c++重写std::map
时间: 2023-07-20 13:39:36 浏览: 76
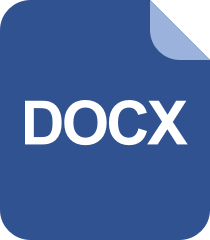
C++ map使用

重写std::map需要实现以下几个关键部分:
1.节点结构体:需要定义一个节点结构体,包含键、值和左右子节点指针。例如:
```c++
template<typename K, typename V>
struct Node {
K key;
V value;
Node<K, V>* left;
Node<K, V>* right;
};
```
2.构造函数:需要定义一个构造函数,初始化根节点为nullptr。例如:
```c++
template<typename K, typename V>
class MyMap {
public:
MyMap():root(nullptr) {}
private:
Node<K, V>* root;
};
```
3.插入操作:需要实现插入操作,将新键值对插入到树中。如果键已经存在,则更新其值。例如:
```c++
template<typename K, typename V>
void MyMap<K, V>::insert(K key, V value) {
if (root == nullptr) {
root = new Node<K, V>{key, value, nullptr, nullptr};
return;
}
Node<K, V>* cur = root;
while (true) {
if (key < cur->key) {
if (cur->left == nullptr) {
cur->left = new Node<K, V>{key, value, nullptr, nullptr};
return;
}
cur = cur->left;
} else if (key > cur->key) {
if (cur->right == nullptr) {
cur->right = new Node<K, V>{key, value, nullptr, nullptr};
return;
}
cur = cur->right;
} else {
cur->value = value;
return;
}
}
}
```
4.查找操作:需要实现查找操作,根据键查找对应的值。如果键不存在,则返回默认值。例如:
```c++
template<typename K, typename V>
V MyMap<K, V>::find(K key, V default_value) {
Node<K, V>* cur = root;
while (cur != nullptr) {
if (key < cur->key) {
cur = cur->left;
} else if (key > cur->key) {
cur = cur->right;
} else {
return cur->value;
}
}
return default_value;
}
```
5.遍历操作:需要实现遍历操作,遍历整棵树,输出所有键值对。例如:
```c++
template<typename K, typename V>
void MyMap<K, V>::traverse() {
traverseHelper(root);
}
template<typename K, typename V>
void MyMap<K, V>::traverseHelper(Node<K, V>* cur) {
if (cur == nullptr) {
return;
}
traverseHelper(cur->left);
std::cout << cur->key << ": " << cur->value << std::endl;
traverseHelper(cur->right);
}
```
以上就是一个简单的重写std::map的示例代码。
阅读全文
相关推荐
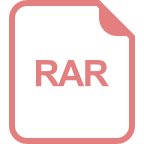
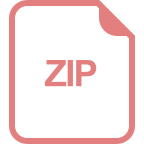


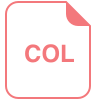
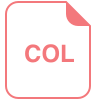
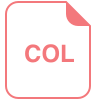



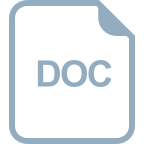
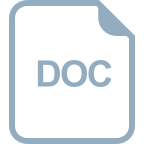
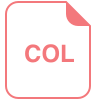
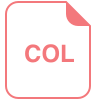
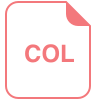
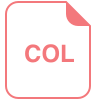
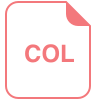
