基于sift算法实现红外图像和可见光图像配准matlab
时间: 2023-08-29 14:12:17 浏览: 71
SIFT算法是一种用于图像特征提取和匹配的经典算法,可以用于红外图像和可见光图像的配准。下面是基于MATLAB实现的红外图像和可见光图像配准流程:
1. 读取红外图像和可见光图像,并将其转换为灰度图像。
2. 对灰度图像进行SIFT特征提取。
3. 使用SIFT特征匹配算法,将红外图像和可见光图像的特征点进行匹配。
4. 使用RANSAC算法去除误匹配的特征点。
5. 根据匹配的特征点,计算红外图像和可见光图像之间的变换矩阵。
6. 使用变换矩阵对红外图像进行变换,使其与可见光图像对应。
7. 可以使用MATLAB中的imshow函数将变换后的红外图像和可见光图像进行对比显示。
下面是MATLAB代码实现:
```matlab
% 读取红外图像和可见光图像
im_ir = imread('ir_image.jpg');
im_rgb = imread('rgb_image.jpg');
% 将图像转换为灰度图像
im_gray_ir = rgb2gray(im_ir);
im_gray_rgb = rgb2gray(im_rgb);
% SIFT特征提取
[f_ir, d_ir] = vl_sift(im2single(im_gray_ir));
[f_rgb, d_rgb] = vl_sift(im2single(im_gray_rgb));
% SIFT特征匹配
[matches, scores] = vl_ubcmatch(d_ir, d_rgb);
% RANSAC去除误匹配的特征点
[~, inliers] = estimateFundamentalMatrix(f_ir(1:2, matches(1,:))', f_rgb(1:2, matches(2,:))', 'Method', 'RANSAC', 'NumTrials', 2000, 'DistanceThreshold', 0.1);
% 计算变换矩阵
tform = estimateGeometricTransform(f_ir(1:2, matches(1,inliers))', f_rgb(1:2, matches(2,inliers))', 'affine');
% 变换红外图像
im_ir_trans = imwarp(im_ir, tform, 'OutputView', imref2d(size(im_rgb)));
% 显示对比图像
figure;
imshowpair(im_rgb, im_ir_trans, 'montage');
title('RGB and IR images registration');
```
相关推荐
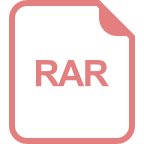














