首页pymysql 增加数据
pymysql 增加数据
时间: 2023-10-23 15:15:07 浏览: 79
使用 PyMySQL 模块可以方便地向 MySQL 数据库中增加数据。下面是一个简单的示例:
```python
import pymysql
# 连接数据库
conn = pymysql.connect(host='localhost', port=3306, user='root', password='password', db='test')
# 创建游标对象
cursor = conn.cursor()
# 执行 SQL 语句
sql = "INSERT INTO student(name, age, gender) VALUES (%s, %s, %s)"
data = ('Tom', 20, 'male')
cursor.execute(sql, data)
# 提交事务
conn.commit()
# 关闭游标和连接
cursor.close()
conn.close()
```
在上述代码中,首先使用 `pymysql.connect()` 方法连接到 MySQL 数据库。然后,使用 `conn.cursor()` 方法创建游标对象。接着,使用 `cursor.execute()` 方法执行 SQL 语句,并传入参数值。最后,使用 `conn.commit()` 方法提交事务,并使用 `cursor.close()` 和 `conn.close()` 方法关闭游标和连接。
阅读全文
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
最新推荐
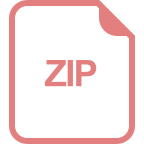
PaddleTS 是一个易用的深度时序建模的Python库,它基于飞桨深度学习框架PaddlePaddle,专注业界领先的深度模型,旨在为领域专家和行业用户提供可扩展的时序建模能力和便捷易用的用户体验
PaddleTS 是一个易用的深度时序建模的Python库,它基于飞桨深度学习框架PaddlePaddle,专注业界领先的深度模型,旨在为领域专家和行业用户提供可扩展的时序建模能力和便捷易用的用户体验。
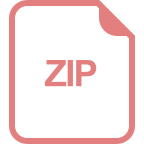
白色大气风格的乐器爱好者网站模板下载.zip
白色大气风格的乐器爱好者网站模板下载.zip
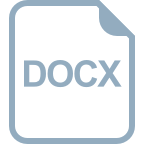
海外派遣员工管理守则.docx
海外派遣员工管理守则
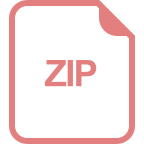
flowable-demo-master
flowable-demo-master
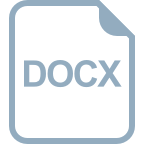
图书管理系统-数据库设计报告.docx
内容概要:本文档详细介绍了一个图书馆管理系统的数据库课程设计。内容涵盖需求分析、数据库设计、SQL实现、前端实现及系统测试等环节。项目旨在支持图书借阅、归还、图书信息管理、用户管理等功能。数据库设计包括三个主要表:用户表(Users)、图书表(Books)和借阅记录表(BorrowRecords)。通过具体示例演示了表的创建、数据插入及查询操作。
适用人群:适合正在学习数据库设计或从事数据库相关工作的学生和技术人员。
使用场景及目标:①学习如何进行需求分析,确定系统的功能和数据需求;②掌握数据库设计方法,绘制ER图并转换为具体的表结构;③编写SQL语句,实现数据的增删改查操作;④实现前端页面,完成与后端的交互;⑤进行系统测试,确保各项功能正常运行。
其他说明:此文档不仅提供了理论知识,还给出了详细的代码示例,非常适合动手实践。建议在学习过程中结合文档中的示例,动手实现数据库设计、SQL操作和前端页面,从而加深对数据库技术的理解。
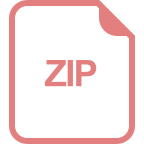
RStudio中集成Connections包以优化数据库连接管理
资源摘要信息:"connections:https"
### 标题解释
标题 "connections:https" 直接指向了数据库连接领域中的一个重要概念,即通过HTTP协议(HTTPS为安全版本)来建立与数据库的连接。在IT行业,特别是数据科学与分析、软件开发等领域,建立安全的数据库连接是日常工作的关键环节。此外,标题可能暗示了一个特定的R语言包或软件包,用于通过HTTP/HTTPS协议实现数据库连接。
### 描述分析
描述中提到的 "connections" 是一个软件包,其主要目标是与R语言的DBI(数据库接口)兼容,并集成到RStudio IDE中。它使得R语言能够连接到数据库,尽管它不直接与RStudio的Connections窗格集成。这表明connections软件包是一个辅助工具,它简化了数据库连接的过程,但并没有改变RStudio的用户界面。
描述还提到connections包能够读取配置,并创建与RStudio的集成。这意味着用户可以在RStudio环境下更加便捷地管理数据库连接。此外,该包提供了将数据库连接和表对象固定为pins的功能,这有助于用户在不同的R会话中持续使用这些资源。
### 功能介绍
connections包中两个主要的功能是 `connection_open()` 和可能被省略的 `c`。`connection_open()` 函数用于打开数据库连接。它提供了一个替代于 `dbConnect()` 函数的方法,但使用完全相同的参数,增加了自动打开RStudio中的Connections窗格的功能。这样的设计使得用户在使用R语言连接数据库时能有更直观和便捷的操作体验。
### 安装说明
描述中还提供了安装connections包的命令。用户需要先安装remotes包,然后通过remotes包的`install_github()`函数安装connections包。由于connections包不在CRAN(综合R档案网络)上,所以需要使用GitHub仓库来安装,这也意味着用户将能够访问到该软件包的最新开发版本。
### 标签解读
标签 "r rstudio pins database-connection connection-pane R" 包含了多个关键词:
- "r" 指代R语言,一种广泛用于统计分析和图形表示的编程语言。
- "rstudio" 指代RStudio,一个流行的R语言开发环境。
- "pins" 指代R包pins,它可能与connections包一同使用,用于固定数据库连接和表对象。
- "database-connection" 指代数据库连接,即软件包要解决的核心问题。
- "connection-pane" 指代RStudio IDE中的Connections窗格,connections包旨在与之集成。
- "R" 代表R语言社区或R语言本身。
### 压缩包文件名称列表分析
文件名称列表 "connections-master" 暗示了一个可能的GitHub仓库名称或文件夹名称。通常 "master" 分支代表了软件包或项目的稳定版或最新版,是大多数用户应该下载和使用的版本。
### 总结
综上所述,connections包是一个专为R语言和RStudio IDE设计的软件包,旨在简化数据库连接过程并提供与Connections窗格的集成。它允许用户以一种更为方便的方式打开和管理数据库连接,而不直接提供与Connections窗格的集成。connections包通过读取配置文件和固定连接对象,增强了用户体验。安装connections包需通过remotes包从GitHub获取最新开发版本。标签信息显示了connections包与R语言、RStudio、数据库连接以及R社区的紧密联系。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
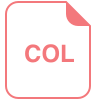
Keil uVision5全面精通指南
# 摘要
Keil uVision5是一个集成开发环境,广泛应用于嵌入式系统的开发。本文从基础入门开始,详细介绍了Keil uVision5的项目管理、配置、编程实践、高级功能及技巧,并通过项目案例分析,深入阐述了其在实际开发中的应用。本篇论文特别注重于项目创建、目标配置、调试环境搭建、性能优化、脚本编程与自动化、高级调试技术,以及通过嵌入式操作系统集成和驱动开发流程的案例分析,展示了Keil uVision5的实用性和高效性。文章还展望了Keil uVision5的未来发展趋势,并提供了社区资源和学习渠道,为读者提供了一个全面掌握Keil uVision5的平台。
# 关键字
Keil u

flink提交给yarn19个全量同步MYsqlCDC的作业,flink的配置参数怎样设置
在Flink中,如果你需要将19个MySQL CDC(Change Data Capture)的全量同步作业提交到YARN上,你需要确保Flink集群和YARN进行了正确的集成,并配置了适当的参数。以下是可能涉及到的一些关键配置:
1. **并行度(Parallelism)**:每个作业的并行度应该设置得足够高,以便充分利用YARN提供的资源。例如,如果你有19个任务,你可以设置总并行度为19或者是一个更大的数,取决于集群规模。
```yaml
parallelism = 19 或者 根据实际资源调整
```
2. **YARN资源配置**:Flink通过`yarn.a
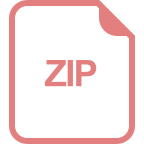
PHP博客旅游的探索之旅
资源摘要信息:"博客旅游"
博客旅游是一个以博客形式分享旅行经验和旅游信息的平台。随着互联网技术的发展和普及,博客作为一种个人在线日志的形式,已经成为人们分享生活点滴、专业知识、旅行体验等的重要途径。博客旅游正是结合了博客的个性化分享特点和旅游的探索性,让旅行爱好者可以记录自己的旅游足迹、分享旅游心得、提供目的地推荐和旅游攻略等。
在博客旅游中,旅行者可以是内容的创造者也可以是内容的消费者。作为创造者,旅行者可以通过博客记录下自己的旅行故事、拍摄的照片和视频、体验和评价各种旅游资源,如酒店、餐馆、景点等,还可以分享旅游小贴士、旅行日程规划等实用信息。作为消费者,其他潜在的旅行者可以通过阅读这些博客内容获得灵感、获取旅行建议,为自己的旅行做准备。
在技术层面,博客平台的构建往往涉及到多种编程语言和技术栈,例如本文件中提到的“PHP”。PHP是一种广泛使用的开源服务器端脚本语言,特别适合于网页开发,并可以嵌入到HTML中使用。使用PHP开发的博客旅游平台可以具有动态内容、用户交互和数据库管理等强大的功能。例如,通过PHP可以实现用户注册登录、博客内容的发布与管理、评论互动、图片和视频上传、博客文章的分类与搜索等功能。
开发一个功能完整的博客旅游平台,可能需要使用到以下几种PHP相关的技术和框架:
1. HTML/CSS/JavaScript:前端页面设计和用户交互的基础技术。
2. 数据库管理:如MySQL,用于存储用户信息、博客文章、评论等数据。
3. MVC框架:如Laravel或CodeIgniter,提供了一种组织代码和应用逻辑的结构化方式。
4. 服务器技术:如Apache或Nginx,作为PHP的运行环境。
5. 安全性考虑:需要实现数据加密、输入验证、防止跨站脚本攻击(XSS)等安全措施。
当创建博客旅游平台时,还需要考虑网站的可扩展性、用户体验、移动端适配、搜索引擎优化(SEO)等多方面因素。一个优质的博客旅游平台,不仅能够提供丰富的内容,还应该注重用户体验,包括页面加载速度、界面设计、内容的易于导航等。
此外,博客旅游平台还可以通过整合社交媒体功能,允许用户通过社交媒体账号登录、分享博客内容到社交网络,从而提升平台的互动性和可见度。
综上所述,博客旅游作为一个结合了旅行分享和在线日志的平台,对于旅行者来说,不仅是一个记录和分享旅行体验的地方,也是一个获取旅行信息、学习旅游知识的重要资源。而对于开发者来说,构建这样一个平台需要运用到多种技术和考虑多个技术细节,确保平台的功能性和用户体验。