#include<math.h> #include<algorithm> #include<iostream> #include<string.h> #define ll long long #define endl "\n" using namespace std; typedef pair<int, int>pll; const int N = 2e5 + 10; pll c[N]; int h[150][150]; int x[N], y[N], dp[5055][5055], ss[N]; int gcd(int a, int b) { return b ? gcd(b, a % b) : a; } ll a[N]; int n; signed main() { int n; cin >> n; dp[1][1] = 1, dp[2][1] = 2; int right = 1; for (int i = 3; i <= n; i++) { for (int j = 1; j <= right; j++) { dp[i][j] = dp[i - 1][j] + dp[i - 2][j]; } for (int j = 1; j <= right; j++) { if (dp[i][j] >= 10) { dp[i][j + 1] += dp[i][j] / 10; dp[i][j] %= 10; if (dp[i][right + 1])right++; } } } for (int i = right; i >= 1; i--) { cout << dp[n][i] ; } return 0; }
时间: 2023-12-20 14:06:01 浏览: 76
这段代码是一个计算斐波那契数列的程序,通过动态规划的方式计算斐波那契数列的第n项的值。
首先,程序从输入中获取一个整数n,表示要计算的斐波那契数列的项数。
然后,程序初始化dp数组,其中dp[i][j]表示斐波那契数列第i项的第j位数字。初始时,dp[1][1]为1,dp[2][1]为2。
接下来,程序通过循环计算dp数组的其他项。对于每个i大于等于3的值,程序通过将前两项的对应位相加得到当前项的值。同时,如果当前位的值大于等于10,就需要进位到下一位。
最后,程序从右往左输出dp数组中第n项的值。
注意,在这段代码中,会存在数组越界的问题。例如,当n=1时,dp[n][i]中的i可能会超出数组的范围。
相关问题
#include <iostream.h> #include <string.h> #include <algorithm.h> #include <bitset.h> #include <vector.h>这五个引用的是什么
这五个引用分别是C++标准库中的头文件,包含了不同的函数和类,具体如下:
1. #include <iostream.h>:数据流输入/输出,包含了cin、cout等对象和相关操作符。
2. #include <string.h>:C语言中处理字符串的头文件,包含了字符串操作函数。
3. #include <algorithm.h>:STL中的头文件,包含了各种算法函数,如排序、查找等。
4. #include <bitset.h>:STL中的头文件,包含了位集合类bitset。
5. #include <vector.h>:STL中的头文件,包含了动态数组类vector。
下列头文件的作用是什么,能分别说明吗#include <iostream> #include "ros/ros.h" #include "std_msgs/String.h" #include "std_msgs/Float32.h" #include <sstream> #include "math.h" #include "can_drive/ICANCmd.h" #include "can_drive/Nav_data_msg.h" #include "can_drive/encoder_vel_msg.h" #include "can_drive/radar_obs_msg.h" #include "can_drive/camera_obs_msg.h" #include "sensor_msgs/Imu.h" #include "can_drive/pwm_cmd_msg.h" #include "can_drive/sensor_states_msg.h" #include <tf/tf.h> #include <bitset> #include <string> #include <algorithm> #include <thread> #include <pthread.h> #include <time.h>
这些头文件是C++编程中常用的库文件,主要作用如下:
- #include <iostream>:用于输入输出流的操作,如输出到控制台等。
- #include "ros/ros.h":ROS机器人操作系统的头文件,用于机器人程序的编写。
- #include "std_msgs/String.h":ROS中的标准消息类型,用于传递字符串类型的消息。
- #include "std_msgs/Float32.h":ROS中的标准消息类型,用于传递浮点型数据。
- #include <sstream>:用于字符串流的操作,如将数字转换为字符串等。
- #include "math.h":数学库文件,提供了许多常用的数学函数。
- #include "can_drive/ICANCmd.h":自定义消息类型,用于控制CAN总线通信。
- #include "can_drive/Nav_data_msg.h":自定义消息类型,用于传递导航数据。
- #include "can_drive/encoder_vel_msg.h":自定义消息类型,用于传递编码器速度数据。
- #include "can_drive/radar_obs_msg.h":自定义消息类型,用于传递雷达障碍物数据。
- #include "can_drive/camera_obs_msg.h":自定义消息类型,用于传递摄像头障碍物数据。
- #include "sensor_msgs/Imu.h":ROS中的标准消息类型,用于传递IMU数据。
- #include "can_drive/pwm_cmd_msg.h":自定义消息类型,用于控制PWM信号输出。
- #include "can_drive/sensor_states_msg.h":自定义消息类型,用于传递各种传感器状态数据。
- #include <tf/tf.h>:ROS中的变换库文件,用于实现坐标系变换。
- #include <bitset>:二进制库文件,提供了位运算的一些常用函数。
- #include <string>:字符串库文件,提供了许多字符串操作的函数。
- #include <algorithm>:STL算法库文件,提供了许多常用的算法函数。
- #include <thread>:C++11中的多线程库文件,用于实现多线程编程。
- #include <pthread.h>:POSIX线程库文件,用于实现多线程编程。
- #include <time.h>:时间库文件,提供了许多时间相关的函数。
阅读全文
相关推荐
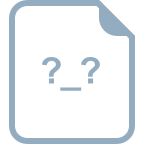
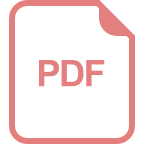
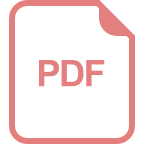














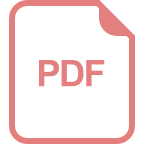