在函数内填入适当内容来完成算法,求链表中第i个结点的指针,若不存在,则返回NULL。
时间: 2023-08-31 18:19:55 浏览: 57
### 回答1:
```c++
ListNode* getIthNode(ListNode* head, int i) {
int count = 1;
ListNode* p = head;
while (p && count != i) {
p = p->next;
count++;
}
return p;
}
```
### 回答2:
首先,在函数的输入参数中需要包含链表的头节点指针和要查找的第i个节点的索引i。
算法如下:
1. 如果传入的头节点指针为空,则链表为空,直接返回NULL。
2. 初始化一个指针p为头节点。
3. 用一个整数count来记录当前遍历到的节点的索引,初始值为1。
4. 进入循环,在循环中判断两个条件:当前count等于传入的i,或者p指针为空。
a. 如果当前count等于传入的i,则找到第i个节点,返回p指针。
b. 如果p指针为空,则链表中不存在第i个节点,返回NULL。
5. 若以上条件均不满足,则继续遍历下一个节点。
a. 将p指针移向下一个节点,即p = p->next。
b. 将count加1,即count++。
6. 重复步骤4-5,直到找到第i个节点或者链表遍历结束。
最后,如果循环结束也没有找到第i个节点,则返回NULL。
以下是算法的C代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
//链表节点结构体定义
struct ListNode {
int data;
struct ListNode* next;
};
struct ListNode* findNode(struct ListNode* head, int i) {
if (head == NULL) {
return NULL;
}
struct ListNode* p = head;
int count = 1;
while (count != i && p != NULL) {
p = p->next;
count++;
}
if (count == i) {
return p;
}
else {
return NULL;
}
}
int main() {
// 创建链表
struct ListNode* head = (struct ListNode*)malloc(sizeof(struct ListNode));
struct ListNode* node1 = (struct ListNode*)malloc(sizeof(struct ListNode));
struct ListNode* node2 = (struct ListNode*)malloc(sizeof(struct ListNode));
struct ListNode* node3 = (struct ListNode*)malloc(sizeof(struct ListNode));
head->data = 1;
head->next = node1;
node1->data = 2;
node1->next = node2;
node2->data = 3;
node2->next = node3;
node3->data = 4;
node3->next = NULL;
// 查找第2个节点
struct ListNode* result = findNode(head, 2);
if (result == NULL) {
printf("不存在第2个节点\n");
}
else {
printf("第2个节点的数据为:%d\n", result->data);
}
free(head);
free(node1);
free(node2);
free(node3);
return 0;
}
```
以上代码中,我创建了一个简单的链表,其中有四个节点,然后我调用`findNode`函数去查找第二个节点,并打印出结果。
### 回答3:
可以使用以下算法来求解链表中第i个结点的指针:
1. 首先,定义一个指针变量p指向链表的头结点。
2. 定义一个变量count并初始化为1,用来计数当前遍历到的结点位置。
3. 通过循环遍历链表,当count小于i时,继续遍历链表,让p指向下一个结点,并将计数器count加1。当count等于i时,表示找到了第i个结点,此时返回p指针。
4. 若循环结束时仍然没有找到第i个结点,则返回NULL。
以下是用C++实现上述算法的示例代码:
```
struct ListNode {
int val;
ListNode* next;
};
ListNode* getNthNode(ListNode* head, int i) {
ListNode* p = head;
int count = 1;
while (p && count < i) {
p = p->next;
count++;
}
if (count == i) {
return p;
} else {
return NULL;
}
}
```
该算法的时间复杂度为O(i),其中i表示要求的结点位置。
相关推荐
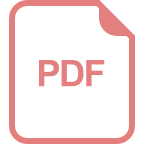
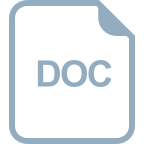
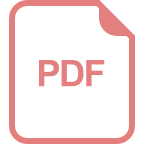














