python实现撤销功能
时间: 2023-09-06 10:05:08 浏览: 304
### 回答1:
在 Python 中实现撤销功能可以通过使用“命令模式”(Command Pattern)来实现。命令模式将操作封装到一个对象中,使得可以将撤销操作作为一个对象存储,并在需要撤销时执行。
以下是一个简单的实现示例:
```python
class Command:
def execute(self):
pass
def undo(self):
pass
class AddCommand(Command):
def __init__(self, data, collection):
self.data = data
self.collection = collection
def execute(self):
self.collection.append(self.data)
def undo(self):
self.collection.remove(self.data)
class Invoker:
def __init__(self):
self.history = []
def execute_command(self, command):
command.execute()
self.history.append(command)
def undo_last_command(self):
if self.history:
self.history.pop().undo()
```
在上面的示例中,`Command` 类是一个抽象类,定义了两个方法 `execute` 和 `undo`,分别用于执行和撤销操作。`AddCommand` 类继承自 `Command`,用于添加数据到集合中。`Invoker` 类用于执行和撤销命令,并维护一个历史记录。可以通过调用 `execute_command` 方法执行一个命令,并将命令添加到历史记录中。可以通过调用 `undo_last_command` 方法撤销上一个命令。
使用示例:
```python
collection = []
invoker = Invoker()
# 添加数据
command1 = AddCommand("data1", collection)
invoker.execute_command(command1)
command2 = AddCommand("data2", collection)
invoker.execute_command(command2)
# 撤销操作
invoker.undo_last_command() # 撤销添加 "data2"
invoker.undo_last_command() # 撤销添加 "data1"
```
在上面的示例中,先创建了一个空集合 `collection`,然后创建了两个 `AddCommand` 对象分别添加数据到集合中。最后通过调用 `undo_last_command` 方法撤销上一个命令,可以撤销添加的数据。
### 回答2:
Python实现撤销功能可以通过使用栈数据结构来实现。下面是一个示例代码:
```
class TextEditor:
def __init__(self):
self.text = ""
self.undo_stack = []
def insert_text(self, new_text):
self.undo_stack.append(self.text)
self.text += new_text
def delete_text(self, length):
if length > len(self.text):
length = len(self.text)
self.undo_stack.append(self.text)
self.text = self.text[:-length]
def undo(self):
if self.undo_stack:
self.text = self.undo_stack.pop()
else:
print("Nothing to undo")
# 使用示例
editor = TextEditor()
editor.insert_text("Hello")
editor.insert_text(" World")
print(editor.text) # 输出: Hello World
editor.delete_text(6)
print(editor.text) # 输出: Hello
editor.undo()
print(editor.text) # 输出: Hello World
editor.undo()
print(editor.text) # 输出: Hello World (因为已经没有撤销操作了)
```
在这个示例代码中,我们使用了两个方法insert_text和delete_text来对text属性进行修改,并将每次修改前的文本内容保存到undo_stack中。而undo方法则是将undo_stack中最近的一个保存的文本内容取出来,并赋值给text属性。如果undo_stack已经为空,则打印"Nothing to undo",表示没有可以撤销的操作了。
### 回答3:
在Python中实现撤销功能可以通过操作栈实现。我们可以使用两个栈,一个用于存储操作记录的栈,另一个用于存储被撤销的操作。
首先,创建一个空的栈,用于存储操作记录。
当用户进行操作时,将操作记录存入栈中。
当用户触发撤销操作时,首先判断操作记录栈是否为空,如果为空则无法进行撤销操作,如果不为空,则从操作记录栈中弹出最新的操作记录,并将其存入被撤销操作栈中。
如果用户希望恢复之前的操作,可以从被撤销操作栈中弹出最新的操作记录,并将其重新存入操作记录栈中。
以下是一个简单的示例代码:
```python
class UndoManager:
def __init__(self):
self.operation_stack = [] # 操作记录栈
self.undo_stack = [] # 被撤销操作栈
def perform_operation(self, operation):
self.operation_stack.append(operation)
def undo(self):
if len(self.operation_stack) > 0:
operation = self.operation_stack.pop()
self.undo_stack.append(operation)
else:
print("无法进行撤销操作,操作记录栈为空")
def redo(self):
if len(self.undo_stack) > 0:
operation = self.undo_stack.pop()
self.operation_stack.append(operation)
else:
print("无法进行恢复操作,被撤销操作栈为空")
```
通过上述代码,我们可以实现对操作的撤销和恢复操作。当用户进行操作时,调用`perform_operation`方法,传入对应的操作记录。当用户触发撤销操作时,调用`undo`方法,会将最新的操作记录从操作记录栈中弹出,并存入被撤销操作栈中。当用户触发恢复操作时,调用`redo`方法,会将最新的被撤销操作记录从被撤销操作栈中弹出,并存入操作记录栈中。
需要注意的是,上述代码只是一个简单的示例,实际应用中可能需要根据具体业务进行修改和扩展。
阅读全文
相关推荐
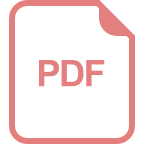
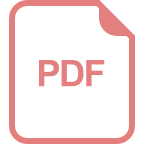
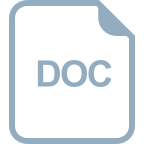



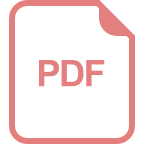
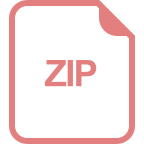
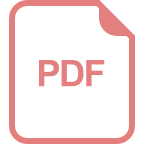
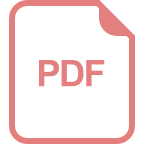
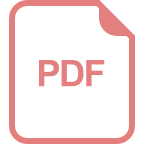
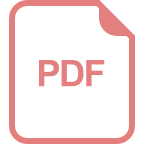
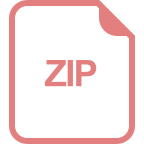
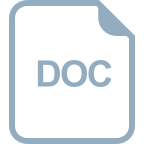
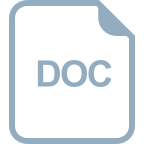
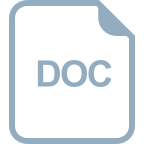

