使用stm32f103rct6控制dht11检测温湿度,请给出代码
时间: 2023-08-26 18:05:03 浏览: 104
以下是使用STM32F103RCT6控制DHT11检测温湿度的示例代码:
```
#include "stm32f10x.h"
// 定义DHT11数据端口和引脚
#define DHT11_PORT GPIOA
#define DHT11_PIN GPIO_Pin_0
// 定义DHT11数据总线电平
#define HIGH 1
#define LOW 0
// 声明DHT11初始化函数
void DHT11_Init(void);
// 声明DHT11读取数据函数
void DHT11_ReadData(uint8_t *pBuffer);
// 延时函数
void Delay_us(uint32_t us);
int main(void)
{
uint8_t buffer[5]; // 存储DHT11返回的5个字节数据
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE); // 使能GPIOA时钟
DHT11_Init(); // 初始化DHT11
while(1)
{
DHT11_ReadData(buffer); // 读取DHT11数据
// TODO: 处理DHT11返回的数据
}
}
void DHT11_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = DHT11_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DHT11_PORT, &GPIO_InitStructure);
GPIO_SetBits(DHT11_PORT, DHT11_PIN); // 设置DHT11数据总线为高电平
Delay_us(20000); // 延时20ms
}
void DHT11_ReadData(uint8_t *pBuffer)
{
uint8_t i, j;
uint8_t temp = 0;
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = DHT11_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DHT11_PORT, &GPIO_InitStructure);
GPIO_SetBits(DHT11_PORT, DHT11_PIN); // 设置DHT11数据总线为高电平
Delay_us(5); // 延时5us
GPIO_ResetBits(DHT11_PORT, DHT11_PIN); // 将DHT11数据总线拉低
Delay_us(20); // 延时20us
GPIO_SetBits(DHT11_PORT, DHT11_PIN); // 将DHT11数据总线拉高
Delay_us(40); // 延时40us
GPIO_InitStructure.GPIO_Pin = DHT11_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DHT11_PORT, &GPIO_InitStructure);
// 等待DHT11响应信号
while(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) == HIGH);
// 等待DHT11回应信号
while(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) == LOW);
// 读取DHT11返回的5个字节数据
for(i = 0; i < 5; i++)
{
for(j = 0; j < 8; j++)
{
// 等待DHT11发送数据位
while(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) == LOW);
// 读取DHT11发送的数据位
Delay_us(30); // 延时30us
temp <<= 1;
if(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) == HIGH)
{
temp |= 0x01;
}
// 等待DHT11发送完数据位
while(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) == HIGH);
}
pBuffer[i] = temp;
temp = 0;
}
}
void Delay_us(uint32_t us)
{
uint32_t i;
for(i = 0; i < us * 8; i++);
}
```
请注意,本示例代码仅供参考,具体实现可能需要根据实际情况进行调整。另外,DHT11的数据传输协议比较特殊,需要注意细节问题。
阅读全文
相关推荐
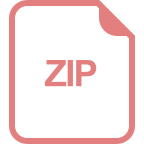



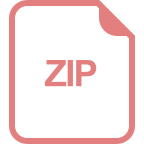
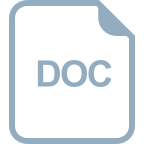






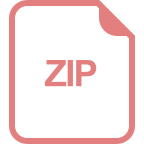



