stm32f103rct6控制dht11实现代码?
时间: 2023-06-07 08:07:20 浏览: 77
以下是基于 stm32f103rct6 控制 dht11 的实现代码,供参考:
```
#include "stdio.h"
#include "stm32f10x.h"
// 定义 DHT11 的引脚
#define DHT11_PORT GPIOF
#define DHT11_PIN GPIO_Pin_8
void DHT11_Delay_us(u16 us)
{
u32 delay_counter = 12 * us;
while (delay_counter --);
}
// DHT11 初始化
void DHT11_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOF, ENABLE);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Pin = DHT11_PIN;
GPIO_Init(DHT11_PORT, &GPIO_InitStructure);
}
// 从DHT11读取一位数据,超时为 100 微秒
u8 DHT11_Read_Bit(void)
{
u8 retry = 0;
while (GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) == RESET)
if (retry ++ > 100) return 0;
DHT11_Delay_us(40);
if (GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) == SET)
{
while (GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) == SET)
;
return 1;
}
else
{
return 0;
}
}
// 从DHT11读取一个字节的数据
u8 DHT11_Read_Byte(void)
{
u8 data = 0;
u8 i;
for (i = 0; i < 8; i ++)
{
data <<= 1;
data |= DHT11_Read_Bit();
}
return data;
}
// 从DHT11读取湿度和温度数据
void DHT11_Read_Data(u8 *temp, u8 *humi)
{
u8 buf[5];
u8 i;
// 主机拉低 DHT11 引脚,等待 18ms 左右
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = DHT11_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DHT11_PORT, &GPIO_InitStructure);
GPIO_ResetBits(DHT11_PORT, DHT11_PIN);
DHT11_Delay_us(20000);
GPIO_SetBits(DHT11_PORT, DHT11_PIN);
// 等待 DHT11 的响应
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(DHT11_PORT, &GPIO_InitStructure);
while (GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) == SET);
if (GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) == RESET)
{
while (GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN) == RESET);
for (i = 0; i < 5; i ++)
{
buf[i] = DHT11_Read_Byte();
}
// 校验
if ((buf[0] + buf[1] + buf[2] + buf[3]) == buf[4])
{
*humi = buf[0];
*temp = buf[2];
}
}
}
int main(void)
{
u8 humi = 0;
u8 temp = 0;
// 初始化
DHT11_Init();
// 读取数据
DHT11_Read_Data(&temp, &humi);
// 在这里进行对读取到的温湿度数据的处理
return 0;
}
```
希望这个代码能够帮助到您,如有任何问题,欢迎随时提出。
相关推荐
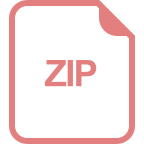
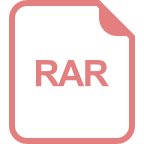














