三分搜索算法的做法是:它先将待查元素x与n/3处的元素比较,然后将x与2n/3处的元素进行比较。比较的结果或者找到x,或者将搜索范围缩小为原来的n/3。请编写c++程序实现算法。
时间: 2023-09-03 11:28:16 浏览: 89
### 回答1:
题目要求实现一种三分搜索算法,具体方法是首先将待查元素x与n/3处的元素比较,然后将x与2n/3处的元素比较。比较的结果要么找到了x,要么缩小了范围为原来的n/3或者n/3。
以下是使用C++编写的实现代码:
```
int ternarySearch(int arr[], int left, int right, int x) {
if (right >= left) {
int mid1 = left + (right - left) / 3;
int mid2 = right - (right - left) / 3;
if (arr[mid1] == x) {
return mid1;
}
if (arr[mid2] == x) {
return mid2;
}
if (x < arr[mid1]) {
// Search the left third
return ternarySearch(arr, left, mid1 - 1, x);
} else if (x > arr[mid2]) {
// Search the right third
return ternarySearch(arr, mid2 + 1, right, x);
} else {
// Search the middle third
return ternarySearch(arr, mid1 + 1, mid2 - 1, x);
}
}
return -1;
}
```
这段代码使用递归的方式实现三分搜索。首先计算出左、右两个中间点,然后分别比较待查元素和这两个点处的元素。如果其中一个点处的元素与待查元素相等,则返回该点的下标。如果待查元素比左侧点处的元素小,则在左侧的第一部分继续递归搜索;如果待查元素比右侧点处的元素大,则在右侧的第三部分继续递归搜索;否则,在中间的第二部分继续递归搜索。
如果全部搜索完毕后,还没有找到目标元素,则返回-1。
### 回答2:
下面是一个用C语言实现三分搜索算法的例子:
```c
#include <stdio.h>
int ternarySearch(int arr[], int l, int r, int x) {
if (r >= l) {
int mid1 = l + (r - l) / 3;
int mid2 = r - (r - l) / 3;
if (arr[mid1] == x) {
return mid1;
}
if (arr[mid2] == x) {
return mid2;
}
if (x < arr[mid1]) {
return ternarySearch(arr, l, mid1 - 1, x);
} else if (x > arr[mid2]) {
return ternarySearch(arr, mid2 + 1, r, x);
} else {
return ternarySearch(arr, mid1 + 1, mid2 - 1, x);
}
}
return -1;
}
int main() {
int arr[] = {1, 3, 5, 7, 9, 11, 13, 15, 17, 19};
int n = sizeof(arr) / sizeof(arr[0]);
int x = 7;
int result = ternarySearch(arr, 0, n - 1, x);
if (result == -1) {
printf("元素 %d 不存在于数组中。\n", x);
} else {
printf("元素 %d 存在于数组的下标 %d 处。\n", x, result);
}
return 0;
}
```
在这个例子中,我们定义了一个名为`ternarySearch`的函数来实现三分搜索算法。该函数接受一个已排序的数组`arr`、搜索范围的起始位置`l`和结束位置`r`,以及要查找的元素`x`。函数首先计算三个中间位置:`mid1 = l + (r - l) / 3`和`mid2 = r - (r - l) / 3`。然后,函数使用条件语句来比较`x`与`mid1`和`mid2`位置处的元素。如果`x`等于其中任一位置处的元素,函数返回相应的位置。否则,如果`x`小于`mid1`处的元素,函数递归地调用自身,将搜索范围缩小到`l`到`mid1 - 1`。如果`x`大于`mid2`处的元素,函数递归地调用自身,将搜索范围缩小到`mid2 + 1`到`r`。否则,如果`x`位于`mid1`和`mid2`之间,函数递归地调用自身,将搜索范围缩小到`mid1 + 1`到`mid2 - 1`。最后,如果搜索范围缩小到只有一个元素且该元素不等于`x`,函数返回-1,表示找不到该元素。
在`main`函数中,我们创建一个示例数组`arr`和一个要查找的元素`x`。然后,我们调用`ternarySearch`函数并打印结果。如果返回值为-1,则打印出元素不存在于数组中的消息;否则,打印出元素存在于数组的下标处的消息。
### 回答3:
以下是一个用C语言实现三分搜索算法的例子:
```c
#include <stdio.h>
int ternarySearch(int arr[], int left, int right, int x) {
if (right >= left) {
int mid1 = left + (right - left) / 3;
int mid2 = right - (right - left) / 3;
if (arr[mid1] == x) {
return mid1;
}
if (arr[mid2] == x) {
return mid2;
}
if (x < arr[mid1]) {
return ternarySearch(arr, left, mid1 - 1, x);
} else if (x > arr[mid2]) {
return ternarySearch(arr, mid2 + 1, right, x);
} else {
return ternarySearch(arr, mid1 + 1, mid2 - 1, x);
}
}
return -1;
}
int main() {
int arr[] = {1, 2, 3, 4, 5, 6, 7, 8, 9};
int n = sizeof(arr) / sizeof(arr[0]);
int x = 5;
int result = ternarySearch(arr, 0, n - 1, x);
if (result == -1) {
printf("元素 %d 不存在于数组中\n", x);
} else {
printf("元素 %d 存在于数组中,位置为 %d\n", x, result);
}
return 0;
}
```
以上代码实现了一个简单的三分搜索算法。在`ternarySearch`函数中,我们将待查找元素与数组的1/3和2/3处的元素进行比较,从而决定下一步的搜索范围。如果找到了待查找元素,则返回其在数组中的位置;如果未找到,则继续递归地在新的搜索范围内进行搜索。在`main`函数中,我们使用刚才实现的算法来查找数组中的元素5,并输出结果。
相关推荐
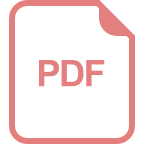
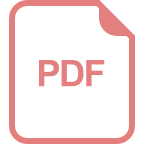
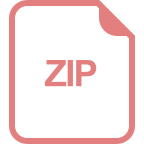













