使用stm32f103编写代码,通过串口通信短信控制灯光开关和亮度
时间: 2024-03-22 09:40:32 浏览: 73
好的,以下是一个简单的代码示例,实现了你的要求:
```C++
#include "stm32f10x.h"
#include <stdio.h>
#define LED_PIN GPIO_Pin_13
#define LED_PORT GPIOC
#define USART_RX_PIN GPIO_Pin_10
#define USART_TX_PIN GPIO_Pin_9
#define USART_GPIO GPIOA
#define USART USART1
#define MAX_CMD_LEN 10
char cmd[MAX_CMD_LEN];
int cmd_pos = 0;
void init_led(void) {
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = LED_PIN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(LED_PORT, &GPIO_InitStructure);
GPIO_SetBits(LED_PORT, LED_PIN);
}
void init_usart(void) {
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = USART_TX_PIN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(USART_GPIO, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = USART_RX_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(USART_GPIO, &GPIO_InitStructure);
USART_InitTypeDef USART_InitStructure;
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART, &USART_InitStructure);
USART_Cmd(USART, ENABLE);
}
void USART1_IRQHandler(void) {
if (USART_GetITStatus(USART, USART_IT_RXNE) != RESET) {
char c = USART_ReceiveData(USART);
if (c == '\r' || c == '\n') {
cmd[cmd_pos] = '\0';
cmd_pos = 0;
if (strcmp(cmd, "LED ON") == 0) {
GPIO_ResetBits(LED_PORT, LED_PIN); // 点亮 LED
printf("LED 点亮了\r\n");
} else if (strcmp(cmd, "LED OFF") == 0) {
GPIO_SetBits(LED_PORT, LED_PIN); // 熄灭 LED
printf("LED 熄灭了\r\n");
} else if (strncmp(cmd, "BRIGHT", 6) == 0) {
int brightness = atoi(cmd + 7);
printf("设置亮度为 %d\r\n", brightness);
// 在这里设置 LED 的亮度,具体实现需要根据实际硬件情况进行编写
} else {
printf("未知命令: %s\r\n", cmd);
}
} else {
cmd[cmd_pos] = c;
cmd_pos++;
if (cmd_pos >= MAX_CMD_LEN) {
cmd_pos = 0;
}
}
}
}
int main(void) {
init_led();
init_usart();
NVIC_InitTypeDef NVIC_InitStructure;
NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
USART_ITConfig(USART, USART_IT_RXNE, ENABLE);
while (1) {
// 主循环中不需要执行任何操作
}
}
```
这段代码使用了 STM32F103 的 USART1 模块,通过串口接收短信命令,并根据命令来控制 LED 灯的开关和亮度。具体实现中,我们使用了 `GPIO_ResetBits` 和 `GPIO_SetBits` 函数来控制 LED 的开关,使用了 `printf` 函数向串口发送调试信息,使用了 `atoi` 函数来将字符串转换为整数。需要注意的是,`atoi` 函数需要包含 `stdlib.h` 头文件。同时,为了防止串口数据接收过程中出现溢出,我们使用了一个固定长度的缓存区 `cmd` 来存储接收到的命令,长度为 `MAX_CMD_LEN`。在串口接收到回车或换行符时,我们将 `cmd` 中的内容解析为命令并执行相应的操作。
阅读全文
相关推荐
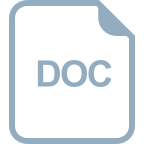
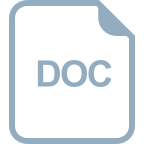
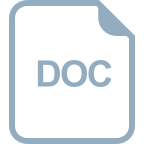

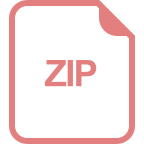
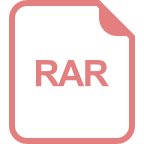
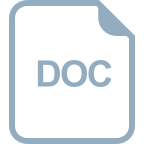

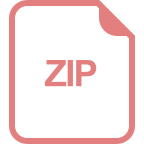
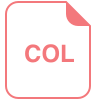
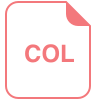
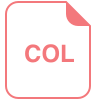
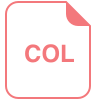
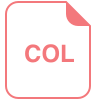
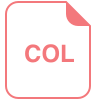
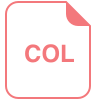
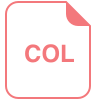
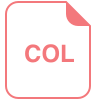
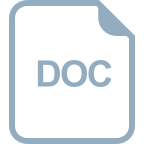