使用python写一段贪吃蛇的代码
时间: 2024-11-20 10:39:59 浏览: 30
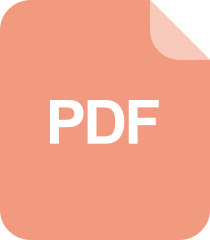
python贪吃蛇游戏代码

编写一个基本的贪吃蛇游戏的Python代码需要一些基础的游戏循环、输入处理、图形显示以及蛇的移动规则。这里是一个简单的命令行版贪吃蛇游戏示例:
```python
import curses
import random
# 初始化窗口
stdscr = curses.initscr()
curses.curs_set(0) # 隐藏光标
# 窗口大小
rows, cols = stdscr.getmaxyx()
snake = [(5, 5), (4, 5), (3, 5)] # 蛇的位置列表
food = (random.randint(1, rows - 2), random.randint(1, cols - 2)) # 食物位置
direction = 'U' # 初始方向为上
while True:
stdscr.clear()
for y in range(rows):
for x in range(cols):
if (x, y) == food:
stdscr.addch(y, x, '*')
elif (x, y) in snake:
stdscr.addch(y, x, '#')
else:
stdscr.addch(y, x, ' ')
# 更新蛇的位置并检查边界
head_x, head_y = snake[0]
if direction == 'U':
head_y -= 1
elif direction == 'D':
head_y += 1
elif direction == 'L':
head_x -= 1
elif direction == 'R':
head_x += 1
if head_x < 0 or head_x >= cols or head_y < 0 or head_y >= rows:
stdscr.addstr("Game Over! Press any key to restart.")
break
snake.insert(0, (head_x, head_y))
if snake[0] == food:
food = (random.randint(1, rows - 2), random.randint(1, cols - 2))
snake.extend([(snake[-1][0], snake[-1][1] + 1),
(snake[-1][0] + 1, snake[-1][1]),
(snake[-1][0] - 1, snake[-1][1])])
else:
tail = snake.pop()
stdscr.addch(tail[1], tail[0], ' ')
# 检查碰撞
for body_part in snake[1:]:
if body_part == head:
stdscr.addstr("Game Over! Press any key to restart.")
break
stdscr.refresh()
c = stdscr.getch() # 获取用户按键
if c in [ord('w'), ord('W')]:
direction = 'U'
elif c in [ord('s'), ord('S')]:
direction = 'D'
elif c in [ord('a'), ord('A')]:
direction = 'L'
elif c in [ord('d'), ord('D')]:
direction = 'R'
curses.endwin()
```
阅读全文
相关推荐
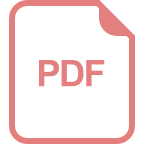
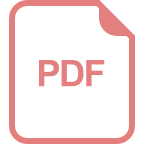










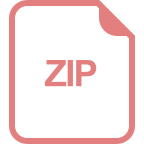
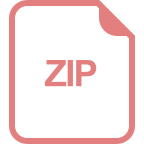
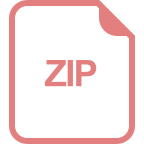
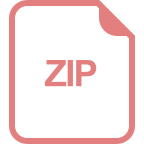
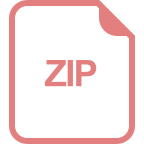