cpp vector<int>的end()
时间: 2023-09-23 19:03:41 浏览: 36
`end()` 是 C++ 容器(如`vector`)的成员函数之一,用于返回指向容器尾部下一个位置(即越界位置)的迭代器。
对于`vector<int>`类型的容器,`end()`函数返回的是一个指向容器中最后一个元素的下一个位置的迭代器。这个迭代器可以用来遍历整个容器,一般用于在循环中判断是否到达了容器的尾部。
以下是一个使用 `end()` 函数来遍历 `vector<int>` 容器的示例代码:
```cpp
#include <iostream>
#include <vector>
int main()
{
std::vector<int> vec = {1, 2, 3, 4, 5};
// 遍历 vector<int> 容器
for (auto it = vec.begin(); it != vec.end(); ++it)
{
std::cout << *it << " ";
}
std::cout << std::endl;
return 0;
}
```
在上面的示例代码中,我们使用`begin()`函数获取指向容器头部的迭代器,使用`end()`函数获取指向容器尾部下一个位置的迭代器。在循环中,我们使用迭代器遍历整个容器,并使用`*`操作符获取当前迭代器指向的元素的值,并输出到控制台上。
相关问题
vector<int>a;
`vector<int> a;`是C++中创建一个名为a的空vector对象的语句,其中`<int>`表示该vector对象只能存储整型数据。可以使用`push_back()`方法向该vector对象中添加元素,例如:
```cpp
vector<int> a;
a.push_back(1);
a.push_back(2);
a.push(3);
```
这将在a中添加三个整数1、2和3。可以使用a.size()`方法获取a中元素的数量,使用`a[i]`访问第i个元素,其中i是一个从0开始的整数索引。可以使用迭代器遍历整个vector对象,例如:
```cpp
vector<int>::iterator it;
for(it=a.begin();it!=a.end();it++){
cout<<*it<<endl;
}
```
这将输出a中的所有元素。需要注意的是,在使用vector对象之前,需要在代码中包含vector头文件,即`#include<vector>`。
vector<vector<int>> sort
vector<vector<int>> sort 是一个函数,而不是一个变量。它可以帮助你对二维向量进行排序。以下是一个示例代码,演示如何使用该函数对二维向量进行排序:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
bool compare(const vector<int>& a, const vector<int>& b) {
// 自定义的比较函数,根据需要进行修改
return a[0] < b[0];
}
int main() {
vector<vector<int>> nums = {{3, 2, 1}, {6, 5, 4}, {9, 8, 7}};
sort(nums.begin(), nums.end(), compare);
for (const auto& row : nums) {
for (const auto& num : row) {
cout << num << " ";
}
cout << endl;
}
return 0;
}
```
这段代码使用 compare 函数作为排序的依据,根据二维向量中每个子向量的第一个元素进行升序排序。你可以根据自己的需求修改 compare 函数来实现不同的排序方式。
相关推荐
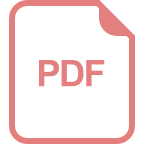
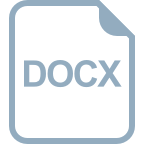
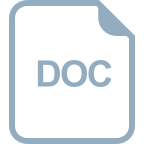












