设计一个算法统计输入字符串中各个不同字符出现的频率并且将结果存入文件(字符串中的合法字符为A~Z这26个字母和0~9这10个数字)用c++语言来实现完整程序
时间: 2024-05-03 18:16:31 浏览: 64
好的,这是一个比较简单的问题,可以使用哈希表来实现。以下是我的建议:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h> //包含 isdigit() 和 isalpha() 函数
#define MAX_LEN 1000 //定义输入字符串的最大长度
#define HASH_SIZE 36 //定义哈希表的大小,即字符集大小
typedef struct node {
char key;
int count;
struct node *next;
} Node;
int hash(char key) {
if (isdigit(key)) {
return key - '0'; //数字的哈希值为0~9
} else {
return key - 'A' + 10; //字母的哈希值为10~35
}
}
void insert(Node *table[], char key) {
int index = hash(key);
Node *p = table[index];
while (p != NULL) {
if (p->key == key) {
p->count++;
return;
}
p = p->next;
}
p = (Node *) malloc(sizeof(Node));
p->key = key;
p->count = 1;
p->next = table[index];
table[index] = p;
}
void print_result(Node *table[]) {
FILE *fp = fopen("result.txt", "w");
for (int i = 0; i < HASH_SIZE; i++) {
Node *p = table[i];
while (p != NULL) {
fprintf(fp, "%c:%d\n", p->key, p->count);
p = p->next;
}
}
fclose(fp);
}
int main() {
char str[MAX_LEN];
Node *table[HASH_SIZE] = {NULL}; //初始化哈希表
printf("请输入一个字符串(只包含A~Z和0~9):");
fgets(str, MAX_LEN, stdin); //从标准输入读入字符串
for (int i = 0; str[i] != '\0'; i++) {
if (isalnum(str[i])) { //判断字符是否为合法字符
insert(table, str[i]);
}
}
print_result(table); //将结果存入文件
return 0;
}
```
这个程序使用链表解决哈希冲突,对于每个输入字符,先判断它是否为合法字符,如果是,则计算它的哈希值,然后在对应的哈希桶中查找该字符是否已经出现过,如果出现过,则将计数器加1,否则插入一个新节点。最后,将哈希表中的每个节点的key和count输出到文件中。
阅读全文
相关推荐
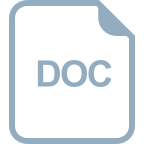
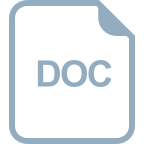
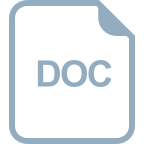















