ffmpeg 编解码h265
时间: 2023-11-20 13:57:37 浏览: 242
要使用FFmpeg进行H.265编码,需要使用x265编码器。以下是使用FFmpeg和x265编码器进行H.265编码的示例命令:
```shell
ffmpeg -i input.mp4 -c:v libx265 -crf 28 -preset medium -c:a aac -b:a 128k output.mp4
```
在这个命令中,我们使用`-c:v`选项指定视频编码器为libx265,使用`-crf`选项指定视频质量,使用`-preset`选项指定编码速度和质量之间的平衡,使用`-c:a`选项指定音频编码器为AAC,使用`-b:a`选项指定音频比特率。
如果要使用FFmpeg进行H.265解码,可以使用以下命令:
```shell
ffmpeg -i input.mp4 -c:v libx265 -c:a copy output.mp4
```
在这个命令中,我们使用`-c:v`选项指定视频编码器为libx265,使用`-c:a`选项指定音频编码器为复制,这将直接从输入文件中复制音频流而不进行重新编码。
相关问题
ffmpeg 硬解码h265
FFmpeg是一个开源的跨平台音视频处理工具,支持包括H.265在内的多种视频编解码。硬解码是利用GPU等硬件加速解码视频,提高解码效率,FFmpeg也支持硬解码。
在FFmpeg中,使用硬解码H.265需要满足以下条件:
1. 安装支持H.265硬解码的硬件设备。
2. 配置FFmpeg时需要添加--enable-cuda或--enable-cuvid选项。
3. 在代码中指定使用cuvid硬解码器。
下面是一个简单的使用示例:
```
AVCodec *codec;
AVCodecContext *codec_ctx;
AVDictionary *opts = NULL;
// 初始化AVCodec和AVCodecContext
codec = avcodec_find_decoder_by_name("hevc_cuvid");
codec_ctx = avcodec_alloc_context3(codec);
av_dict_set(&opts, "threads", "auto", 0);
avcodec_open2(codec_ctx, codec, &opts);
// 解码视频帧
AVPacket pkt;
av_init_packet(&pkt);
pkt.data = inbuf;
pkt.size = insize;
int ret = avcodec_send_packet(codec_ctx, &pkt);
if (ret < 0) {
// 错误处理
}
AVFrame *frame = av_frame_alloc();
ret = avcodec_receive_frame(codec_ctx, frame);
if (ret < 0) {
// 错误处理
}
// 清理资源
av_packet_unref(&pkt);
av_frame_free(&frame);
avcodec_close(codec_ctx);
avcodec_free_context(&codec_ctx);
```
ffmpeg用c++实现h265编解码
要使用FFmpeg库在C++中实现H.265编解码,您可以按照以下步骤进行操作:
1. 首先,确保您已经安装了FFmpeg库。您可以从FFmpeg的官方网站(https://www.ffmpeg.org/)下载源代码并进行编译安装。
2. 在C++代码中包含必要的头文件和链接FFmpeg库。例如:
```cpp
extern "C" {
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/imgutils.h>
#include <libswscale/swscale.h>
}
```
3. 初始化FFmpeg库,注册编解码器和格式。您可以使用`av_register_all()`函数来完成初始化。
```cpp
av_register_all();
```
4. 打开输入文件并获取流信息。
```cpp
AVFormatContext* formatContext = avformat_alloc_context();
if (avformat_open_input(&formatContext, inputFilePath, nullptr, nullptr) != 0) {
// 处理打开文件错误
}
if (avformat_find_stream_info(formatContext, nullptr) < 0) {
// 处理获取流信息错误
}
int videoStreamIndex = -1;
for (int i = 0; i < formatContext->nb_streams; i++) {
if (formatContext->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
videoStreamIndex = i;
break;
}
}
if (videoStreamIndex == -1) {
// 找不到视频流
}
AVCodecParameters* codecParameters = formatContext->streams[videoStreamIndex]->codecpar;
```
5. 查找并打开解码器。
```cpp
AVCodec* codec = avcodec_find_decoder(codecParameters->codec_id);
if (codec == nullptr) {
// 处理找不到解码器错误
}
AVCodecContext* codecContext = avcodec_alloc_context3(codec);
if (avcodec_parameters_to_context(codecContext, codecParameters) < 0) {
// 处理从参数设置解码器上下文错误
}
if (avcodec_open2(codecContext, codec, nullptr) < 0) {
// 处理打开解码器错误
}
```
6. 创建AVFrame来存储解码后的图像帧。
```cpp
AVFrame* frame = av_frame_alloc();
if (frame == nullptr) {
// 处理分配帧内存错误
}
```
7. 创建AVPacket来存储压缩的数据包。
```cpp
AVPacket* packet = av_packet_alloc();
if (packet == nullptr) {
// 处理分配包内存错误
}
```
8. 读取并解码每个数据包,然后进行相应处理。
```cpp
while (av_read_frame(formatContext, packet) >= 0) {
if (packet->stream_index == videoStreamIndex) {
int ret = avcodec_send_packet(codecContext, packet);
if (ret < 0) {
// 处理发送数据包到解码器错误
}
while (ret >= 0) {
ret = avcodec_receive_frame(codecContext, frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
break;
}
else if (ret < 0) {
// 处理从解码器接收帧错误
}
// 在这里进行解码后的图像帧的处理
av_frame_unref(frame);
}
}
av_packet_unref(packet);
}
```
9. 释放资源。
```cpp
av_packet_free(&packet);
av_frame_free(&frame);
avcodec_free_context(&codecContext);
avformat_close_input(&formatContext);
```
以上是一个简要的示例,用于了解如何使用FFmpeg库在C++中实现H.265编解码。请注意,实际的编解码过程可能会更加复杂,并且可能需要根据您的具体需求进行更多的参数设置和错误处理。
阅读全文
相关推荐
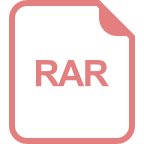
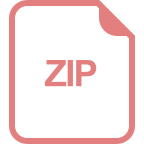
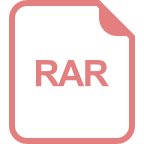













