std::vector 常用函数
时间: 2024-01-05 07:21:06 浏览: 34
std::vector是C++标准库中的一个容器,提供了丰富的成员函数和操作符,用于方便地对元素进行访问、插入、删除和修改等操作。以下是std::vector常用的一些函数:
1. push_back:将元素添加到vector的末尾。
```cpp
std::vector<int> vec;
vec.push_back(10); // 添加元素10到vector的末尾
```
2. pop_back:删除vector的最后一个元素。
```cpp
std::vector<int> vec = {10, 20, 30};
vec.pop_back(); // 删除最后一个元素,vec变为{10, 20}
```
3. size:返回vector中元素的个数。
```cpp
std::vector<int> vec = {10, 20, 30};
int size = vec.size(); // size的值为3
```
4. empty:检查vector是否为空。
```cpp
std::vector<int> vec;
bool isEmpty = vec.empty(); // isEmpty的值为true
```
5. clear:清空vector中的所有元素。
```cpp
std::vector<int> vec = {10, 20, 30};
vec.clear(); // 清空vector,vec变为空
```
6. at:访问指定位置的元素,带有边界检查。
```cpp
std::vector<int> vec = {10, 20, 30};
int element = vec.at(1); // element的值为20
```
7. front:返回vector的第一个元素。
```cpp
std::vector<int> vec = {10, 20, 30};
int firstElement = vec.front(); // firstElement的值为10
```
8. back:返回vector的最后一个元素。
```cpp
std::vector<int> vec = {10, 20, 30};
int lastElement = vec.back(); // lastElement的值为30
```
9. erase:删除指定位置或指定范围内的元素。
```cpp
std::vector<int> vec = {10, 20, 30, 40, 50};
vec.erase(vec.begin() + 2); // 删除第3个元素,vec变为{10, 20, 40, 50}
vec.erase(vec.begin() + 1, vec.begin() + 3); // 删除第2个和第3个元素,vec变为{10, 50}
```
10. insert:在指定位置插入元素。
```cpp
std::vector<int> vec = {10, 20, 30};
vec.insert(vec.begin() + 1, 15); // 在第2个位置插入元素15,vec变为{10, 15, 20, 30}
```
相关推荐
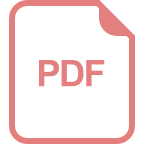
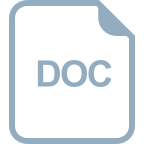
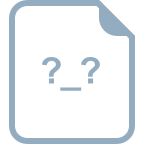














