template <typename T> int compare<std::vector<T>>(const std::vector<T> &lhs, const std::vector<T> &rhs);有什么错误
时间: 2023-09-13 09:12:30 浏览: 45
在模板函数的函数名中,应该使用模板参数名 `T`,而不是具体的类型 `std::vector<T>`。因此,正确的写法应该是:
```
template <typename T>
int compare(const std::vector<T> &lhs, const std::vector<T> &rhs);
```
或者使用 `typename` 的简化形式:
```
template <class T>
int compare(const std::vector<T> &lhs, const std::vector<T> &rhs);
```
这样定义的模板函数可以比较两个 vector 容器内的元素是否相等。
相关问题
int compare<std::vector<T>>(const std::vector<T> &lhs, const std::vector<T> &rhs);
在模板函数的函数名中,使用尖括号 `<>` 显式指定模板参数是错误的语法。在模板函数的定义中,应该使用 `template <typename T>` 或 `template <class T>` 语法来声明模板参数,而不需要显式指定模板参数类型。
因此,正确的写法应该是:
```
template <typename T>
int compare(const std::vector<T> &lhs, const std::vector<T> &rhs);
```
或者使用 `typename` 的简化形式:
```
template <class T>
int compare(const std::vector<T> &lhs, const std::vector<T> &rhs);
```
这样定义的模板函数可以比较两个 vector 容器内的元素是否相等。
/* 请在此处分别编写Matrix类、重载operator*函数、printMatrix函数 */ template class Matrix { public: Matrix(int row, int column); Matrix(int row, int column, const T& value); Vector& operator[](int index); Vector operator[](int index) const; int getRows() const; int getColumns() const; private: Vector<Vector*> rows; // 存放矩阵元素 }; template Matrix::Matrix(int row, int column) : rows(row) { for (int i = 0; i < row; i++) { rows[i] = new Vector(column); } } template Matrix::Matrix(int row, int column, const T& value) :rows(row) { for (int i = 0; i < row; i++) { rows[i] = new Vector(column, value); } } template Vector& Matrix::operator[](int index) { return *rows[index]; } template Vector Matrix::operator[](int index) const { return *rows[index]; } template int Matrix::getRows() const{ return rows.getSize(); } template int Matrix::getColumns() const{ return rows[0]->getSize(); } template Matrix operator*(const Matrix& lhs, const Matrix& rhs) { Matrix res(lhs.getRows(), rhs.getColumns(), 0); for (int i = 0; i < lhs.getRows(); i++) { for (int j = 0; j < rhs.getColumns(); j++) { for (int k = 0; k < lhs.getColumns(); k++) { res[i][j] += lhs[i][k] * rhs[k][j]; } } } return res; } template void printMatrix(const Matrix& m) { for (int i = 0; i < m.getRows(); i++) { for (int j = 0; j < m.getColumns(); j++) { cout << setw(4) << setfill(' ') << m[i][j]; } } }修复代码
修复后的代码如下:
```
#include <iostream>
#include <iomanip>
#include "Vector.h"
template <typename T>
class Matrix {
public:
Matrix(int row, int column);
Matrix(int row, int column, const T& value);
Vector<T>& operator[](int index);
Vector<T> operator[](int index) const;
int getRows() const;
int getColumns() const;
private:
Vector<Vector<T>*> rows; // 存放矩阵元素
};
template <typename T>
Matrix<T>::Matrix(int row, int column) : rows(row) {
for (int i = 0; i < row; i++) {
rows[i] = new Vector<T>(column);
}
}
template <typename T>
Matrix<T>::Matrix(int row, int column, const T& value) :rows(row) {
for (int i = 0; i < row; i++) {
rows[i] = new Vector<T>(column, value);
}
}
template <typename T>
Vector<T>& Matrix<T>::operator[](int index) {
return *rows[index];
}
template <typename T>
Vector<T> Matrix<T>::operator[](int index) const {
return *rows[index];
}
template <typename T>
int Matrix<T>::getRows() const {
return rows.getSize();
}
template <typename T>
int Matrix<T>::getColumns() const {
return rows[0]->getSize();
}
template <typename T>
Matrix<T> operator*(const Matrix<T>& lhs, const Matrix<T>& rhs) {
Matrix<T> res(lhs.getRows(), rhs.getColumns(), 0);
for (int i = 0; i < lhs.getRows(); i++) {
for (int j = 0; j < rhs.getColumns(); j++) {
for (int k = 0; k < lhs.getColumns(); k++) {
res[i][j] += lhs[i][k] * rhs[k][j];
}
}
}
return res;
}
template <typename T>
void printMatrix(const Matrix<T>& m) {
for (int i = 0; i < m.getRows(); i++) {
for (int j = 0; j < m.getColumns(); j++) {
std::cout << std::setw(4) << std::setfill(' ') << m[i][j];
}
std::cout << std::endl;
}
}
```
相关推荐
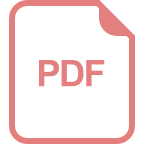
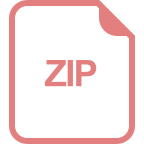







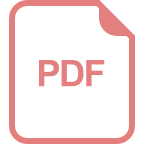
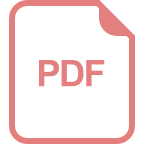
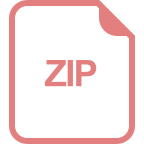
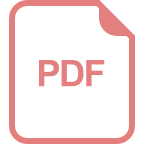
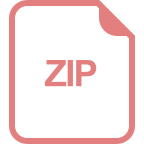
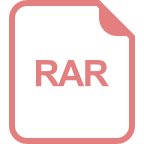
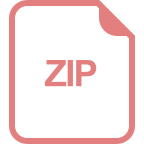