给定一棵用二叉链表表示的二叉树,其根指针为root,试写出求二叉树的深度的算法。c++
时间: 2024-12-16 21:14:02 浏览: 17
为了计算给定二叉链表表示的二叉树的深度,你可以使用递归或者广度优先搜索(BFS)。这里提供两种常见的方法:
**1. 递归方法:**
```cpp
class Solution {
public:
int depthOfBinaryTree(TreeNode* root) {
// 基础情况:空树的深度为0
if (root == nullptr) return 0;
// 计算左子树和右子树的深度,并取较大者加1作为当前树的深度
int leftDepth = depthOfBinaryTree(root->left);
int rightDepth = depthOfBinaryTree(root->right);
// 返回当前节点的最大深度
return std::max(leftDepth, rightDepth) + 1;
}
};
```
**2. 广度优先搜索(BFS)方法:**
```cpp
class Solution {
private:
vector<int> queue;
public:
int depthOfBinaryTree(TreeNode* root) {
if (root == nullptr) return 0;
queue.push_back(0); // 根节点的深度设为0
int depth = 0;
while (!queue.empty()) {
int size = queue.size();
for (int i = 0; i < size; ++i) {
TreeNode* node = queue.front(); // 取出队首节点
queue.pop_front();
// 如果节点存在左右子节点,则继续添加它们的深度到队列
if (node->left) queue.push_back(node->left->val + depth);
if (node->right) queue.push_back(node->right->val + depth);
// 更新深度为当前层节点的数量
depth = std::max(depth, queue.back());
}
}
return depth;
}
};
```
以上两种方法都可以有效计算二叉树的深度。递归方法更简洁直观,但可能会有栈溢出的问题;而BFS方法利用队列存储待访问的节点,空间复杂度较低,适合处理大量数据。
阅读全文
相关推荐
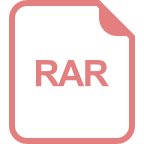

















