二叉树二叉链表存储结构如下:typedef struct BiNodel TElemType data;structBiNode}BiTNode,*BiTree; *Ichild,*rchild;//左右孩子指针 请编写算法求二叉树T中度为2的结点个数。
时间: 2024-11-25 22:18:00 浏览: 13
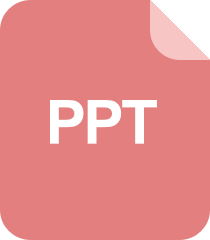
二叉树的二叉链表存储表示-c版本数据结构(严老师)
为了计算给定二叉树 `T` 中度为2的节点数,我们可以创建一个辅助函数,递归地遍历每个节点。`degreeTwoNodes` 函数会检查当前节点是否度为2,并增加计数。如果当前节点有左孩子和右孩子(`lChild` 和 `rChild` 非空),那么它就是度为2的节点。对于左孩子和右孩子的递归调用,我们需要排除掉它们,因为这些节点已经包含在我们正在统计的子树中。
下面是相应的 C++ 算法:
```cpp
int degreeTwoNodes(BiTreeNode* root) {
if (root == nullptr) {
return 0; // 空节点不算
}
int leftDegree = (root->lChild != nullptr) ? 1 : 0;
int rightDegree = (root->rChild != nullptr) ? 1 : 0;
if (leftDegree == 1 && rightDegree == 1) { // 当前节点度为2
return 1 + degreeTwoNodes(root->lChild) + degreeTwoNodes(root->rChild); // 向下递归,加上子树中的度为2节点
} else {
return degreeTwoNodes(root->lChild) + degreeTwoNodes(root->rChild); // 如果不是度为2,仅向下递归
}
}
int getDegreeTwoCount(BiTTree T) {
return degreeTwoNodes(T); // 调用主函数获取整个树中度为2的节点总数
}
```
阅读全文
相关推荐
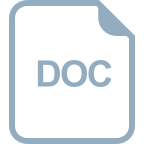
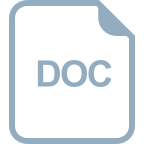
















