3.参照例子15编一个体现 MVC 结构的 GUI 程序。首先编写一个封装梯形类,然后编写一个窗口,要求窗口使用3个文本框和一个文本区为梯形对象中的数据提供视图,其中3个文本框用来显示和更新梯形对象的上底、下底和高,文本区对象用来显示梯形的面积。在窗口中有一个按钮,用户单击该按钮后,程序用3个文本框中的数据分别作为梯形对象的上底、下底和高,并将计算出的梯形的面积显示在文本区中。
时间: 2024-02-22 11:55:12 浏览: 46
以下是一个简单的 Java 程序,实现了上述要求:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
class Trapezoid {
private double upperBase, lowerBase, height;
public Trapezoid(double upperBase, double lowerBase, double height) {
this.upperBase = upperBase;
this.lowerBase = lowerBase;
this.height = height;
}
public double getArea() {
return (upperBase + lowerBase) * height / 2;
}
public double getUpperBase() {
return upperBase;
}
public void setUpperBase(double upperBase) {
this.upperBase = upperBase;
}
public double getLowerBase() {
return lowerBase;
}
public void setLowerBase(double lowerBase) {
this.lowerBase = lowerBase;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
}
class TrapezoidView extends JPanel {
private Trapezoid trapezoid;
private JTextField txtUpperBase, txtLowerBase, txtHeight;
private JTextArea txtArea;
public TrapezoidView(Trapezoid trapezoid) {
this.trapezoid = trapezoid;
setLayout(new BorderLayout());
JPanel topPanel = new JPanel();
topPanel.setLayout(new GridLayout(1, 6));
topPanel.add(new JLabel("上底"));
txtUpperBase = new JTextField(String.valueOf(trapezoid.getUpperBase()));
topPanel.add(txtUpperBase);
topPanel.add(new JLabel("下底"));
txtLowerBase = new JTextField(String.valueOf(trapezoid.getLowerBase()));
topPanel.add(txtLowerBase);
topPanel.add(new JLabel("高"));
txtHeight = new JTextField(String.valueOf(trapezoid.getHeight()));
topPanel.add(txtHeight);
add(topPanel, BorderLayout.NORTH);
txtArea = new JTextArea(String.valueOf(trapezoid.getArea()));
txtArea.setEditable(false);
add(txtArea, BorderLayout.CENTER);
}
public void update() {
trapezoid.setUpperBase(Double.parseDouble(txtUpperBase.getText()));
trapezoid.setLowerBase(Double.parseDouble(txtLowerBase.getText()));
trapezoid.setHeight(Double.parseDouble(txtHeight.getText()));
txtArea.setText(String.valueOf(trapezoid.getArea()));
}
}
class TrapezoidController implements ActionListener {
private TrapezoidView view;
public TrapezoidController(TrapezoidView view) {
this.view = view;
}
public void actionPerformed(ActionEvent e) {
view.update();
}
}
public class TrapezoidGUI extends JFrame {
public TrapezoidGUI() {
super("梯形计算器");
setLayout(new BorderLayout());
Trapezoid trapezoid = new Trapezoid(0, 0, 0);
TrapezoidView view = new TrapezoidView(trapezoid);
add(view, BorderLayout.NORTH);
JPanel bottomPanel = new JPanel();
bottomPanel.setLayout(new FlowLayout());
JButton btnCalculate = new JButton("计算面积");
btnCalculate.addActionListener(new TrapezoidController(view));
bottomPanel.add(btnCalculate);
add(bottomPanel, BorderLayout.SOUTH);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
pack();
setVisible(true);
}
public static void main(String[] args) {
new TrapezoidGUI();
}
}
```
这个程序使用了 MVC(Model-View-Controller)结构,将梯形的数据模型、窗口的视图和事件控制器分别封装成了三个类。在窗口的视图类 TrapezoidView 中,我们使用了 BorderLayout 布局和多个 JPanel 和 JLabel、JTextField、JTextArea 等组件,来展示梯形的上底、下底、高和面积。在事件控制器类 TrapezoidController 中,我们使用了 actionPerformed 方法来处理按钮的单击事件,在事件处理函数中,我们调用视图对象的 update 方法来更新梯形的数据模型,并用新的数据计算出梯形的面积,最后更新窗口中的 JTextArea 组件来显示计算结果。在主函数中,我们创建了一个 TrapezoidGUI 对象来启动程序。
相关推荐
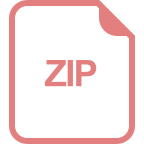














