yuv[:, :, 1] = cv2.resize(yuv[:, :, 1], (yuv.shape[1]//2, yuv.shape[0]//2), interpolation=cv2.INTER_LINEAR) yuv[:, :, 2] = cv2.resize(yuv[:, :, 2], (yuv.shape[1]//2, yuv.shape[0]//2), interpolation=cv2.INTER_LINEAR)出现ValueError: could not broadcast input array from shape (109,89) into shape (218,178)
时间: 2024-02-02 18:03:09 浏览: 120
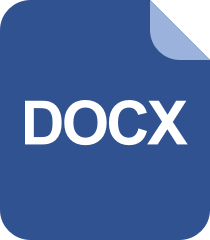
matlab 编程:彩色图转灰度图.docx
这个错误提示表明,你正在尝试将一个大小为 (109, 89) 的数组广播到一个大小为 (218, 178) 的数组中,导致形状不兼容。这个问题通常是由于下采样的输出形状不正确引起的。
下采样操作应该会将图像尺寸减小为原来的一半或者四分之一,因此,你需要确保下采样的输出形状与输入形状成比例。在你的代码中,你正在将U和V通道的形状减半,但是可能存在四舍五入的问题,导致输出形状与输入形状不匹配。
为了解决这个问题,你可以尝试使用下采样函数的参数来确保输出形状正确。例如,你可以使用OpenCV库中的resize函数来进行下采样操作,如下所示:
```python
yuv[:, :, 1] = cv2.resize(yuv[:, :, 1], (yuv.shape[1]//2, yuv.shape[0]//2), interpolation=cv2.INTER_LINEAR)
yuv[:, :, 2] = cv2.resize(yuv[:, :, 2], (yuv.shape[1]//2, yuv.shape[0]//2), interpolation=cv2.INTER_LINEAR)
```
在这个代码中,你正在将U和V通道的形状减半,并使用了OpenCV的resize函数来进行下采样操作。通过这种方式,你可以确保输出形状与输入形状成比例,避免了形状不兼容的问题。
如果还有问题,请提供更多的代码和错误信息,以便更好地帮助你解决问题。
阅读全文
相关推荐
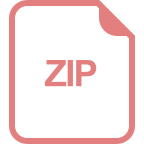
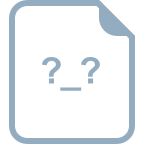















