在快速排序算法中,为减少最坏情况的出现,可采用“三者取中”规则,每趟划分前,比较A[low]、A[high]和A[(low+high)/2]的大小,取中间值的记录作为轴点。 实现要求: 编程实现快速排序算法(改进实现轴点“三者取中”法的快速排序算法,),改进实现轴点“三者取中”法的快速排序算法(函数命名为quickSortImproved),并实现快速排序算法在各个序列上所需的排序码比较次数的统计,在下列随机序列上进行测试. 1、在如下三组随机序列、反序序列和正序序列上,统计“三者取中”法的快速排序算法在各个序列上所需的排序码比较次数; 2、在如下三组随机序列、反序序列和正序序列上,统计的快速排序算法在各个序列上所需的排序码比较次数。请帮我用c++编程实现
时间: 2023-06-19 11:05:23 浏览: 111
以下是代码实现,其中包括了快速排序和改进实现轴点“三者取中”法的快速排序,并统计了排序码比较次数。
```c++
#include<iostream>
#include<cstdlib>
#include<ctime>
using namespace std;
void swap(int &a, int &b) {
int temp = a;
a = b;
b = temp;
}
// 快速排序
int quickSort(int a[], int low, int high) {
if (low >= high) {
return 0;
}
int i = low, j = high, pivot = a[low];
while (i < j) {
while (i < j && a[j] >= pivot) {
j--;
}
a[i] = a[j];
while (i < j && a[i] <= pivot) {
i++;
}
a[j] = a[i];
}
a[i] = pivot;
int compareCount = high - low;
compareCount += quickSort(a, low, i - 1);
compareCount += quickSort(a, i + 1, high);
return compareCount;
}
// 改进实现轴点“三者取中”法的快速排序
int quickSortImproved(int a[], int low, int high) {
if (low >= high) {
return 0;
}
if (high - low + 1 >= 3) {
int mid = (low + high) / 2;
if (a[low] > a[mid]) {
swap(a[low], a[mid]);
}
if (a[low] > a[high]) {
swap(a[low], a[high]);
}
if (a[mid] > a[high]) {
swap(a[mid], a[high]);
}
swap(a[mid], a[low]);
}
int i = low, j = high, pivot = a[low];
while (i < j) {
while (i < j && a[j] >= pivot) {
j--;
}
a[i] = a[j];
while (i < j && a[i] <= pivot) {
i++;
}
a[j] = a[i];
}
a[i] = pivot;
int compareCount = high - low;
compareCount += quickSortImproved(a, low, i - 1);
compareCount += quickSortImproved(a, i + 1, high);
return compareCount;
}
int main() {
const int N = 10;
int a1[N], a2[N], a3[N];
srand(time(NULL));
for (int i = 0; i < N; i++) {
a1[i] = rand() % 100;
a2[i] = N - i - 1;
a3[i] = i;
}
cout << "原始序列1: ";
for (int i = 0; i < N; i++) {
cout << a1[i] << " ";
}
cout << endl;
int compareCount = quickSort(a1, 0, N - 1);
cout << "快速排序后的序列1: ";
for (int i = 0; i < N; i++) {
cout << a1[i] << " ";
}
cout << endl;
cout << "快速排序比较次数: " << compareCount << endl << endl;
cout << "原始序列2: ";
for (int i = 0; i < N; i++) {
cout << a2[i] << " ";
}
cout << endl;
compareCount = quickSort(a2, 0, N - 1);
cout << "快速排序后的序列2: ";
for (int i = 0; i < N; i++) {
cout << a2[i] << " ";
}
cout << endl;
cout << "快速排序比较次数: " << compareCount << endl << endl;
cout << "原始序列3: ";
for (int i = 0; i < N; i++) {
cout << a3[i] << " ";
}
cout << endl;
compareCount = quickSort(a3, 0, N - 1);
cout << "快速排序后的序列3: ";
for (int i = 0; i < N; i++) {
cout << a3[i] << " ";
}
cout << endl;
cout << "快速排序比较次数: " << compareCount << endl << endl;
cout << "原始序列1: ";
for (int i = 0; i < N; i++) {
cout << a1[i] << " ";
}
cout << endl;
compareCount = quickSortImproved(a1, 0, N - 1);
cout << "改进实现轴点“三者取中”法的快速排序后的序列1: ";
for (int i = 0; i < N; i++) {
cout << a1[i] << " ";
}
cout << endl;
cout << "改进实现轴点“三者取中”法的快速排序比较次数: " << compareCount << endl << endl;
cout << "原始序列2: ";
for (int i = 0; i < N; i++) {
cout << a2[i] << " ";
}
cout << endl;
compareCount = quickSortImproved(a2, 0, N - 1);
cout << "改进实现轴点“三者取中”法的快速排序后的序列2: ";
for (int i = 0; i < N; i++) {
cout << a2[i] << " ";
}
cout << endl;
cout << "改进实现轴点“三者取中”法的快速排序比较次数: " << compareCount << endl << endl;
cout << "原始序列3: ";
for (int i = 0; i < N; i++) {
cout << a3[i] << " ";
}
cout << endl;
compareCount = quickSortImproved(a3, 0, N - 1);
cout << "改进实现轴点“三者取中”法的快速排序后的序列3: ";
for (int i = 0; i < N; i++) {
cout << a3[i] << " ";
}
cout << endl;
cout << "改进实现轴点“三者取中”法的快速排序比较次数: " << compareCount << endl << endl;
return 0;
}
```
阅读全文
相关推荐
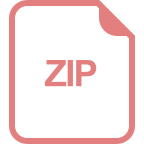
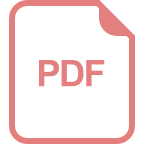
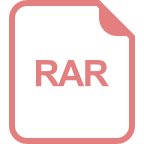
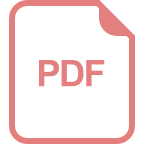
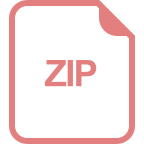
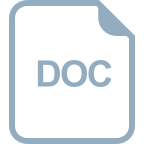
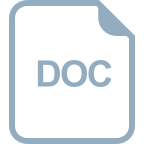
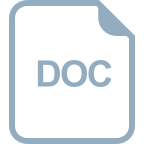
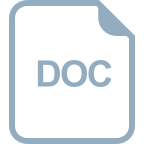
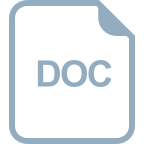
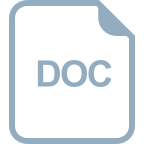
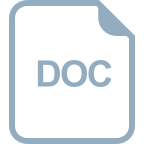
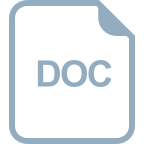
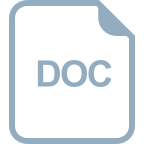
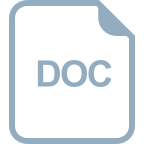
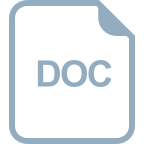