linux 下 cpp 向串口发送命令(16进制数) 并接收串口返回数据(16进制数)
时间: 2023-08-30 15:04:24 浏览: 85
在Linux下,你可以使用C++的串口通信库来实现向串口发送命令并接收返回数据。以下是一个简单的代码示例:
```cpp
#include <iostream>
#include <cstring>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
using namespace std;
int main()
{
// 打开串口
int fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY);
if (fd < 0)
{
cout << "Failed to open serial port!" << endl;
return 1;
}
// 配置串口参数
struct termios options;
tcgetattr(fd, &options);
options.c_cflag = B9600 | CS8 | CLOCAL | CREAD; // 波特率为9600,数据位为8,无校验位,无流控制
options.c_iflag = IGNPAR | ICRNL;
options.c_oflag = 0;
options.c_lflag = 0;
tcflush(fd, TCIFLUSH);
tcsetattr(fd, TCSANOW, &options);
// 发送命令
unsigned char cmd[] = {0x01, 0x02, 0x03}; // 发送的命令
int bytesWritten = write(fd, cmd, sizeof(cmd));
if (bytesWritten < 0)
{
cout << "Failed to send command!" << endl;
close(fd);
return 1;
}
// 接收返回数据
unsigned char buf[1024];
int bytesRead = read(fd, buf, sizeof(buf));
if (bytesRead < 0)
{
cout << "Failed to receive data!" << endl;
close(fd);
return 1;
}
// 输出接收到的数据
for (int i = 0; i < bytesRead; i++)
{
printf("%02X ", buf[i]); // 以16进制格式输出
}
cout << endl;
// 关闭串口
close(fd);
return 0;
}
```
在上面的代码中,我们首先使用`open`函数打开串口,然后使用`tcgetattr`和`tcsetattr`函数配置串口参数。接着,我们使用`write`函数向串口发送命令,使用`read`函数接收串口返回的数据,并最终使用`close`函数关闭串口。注意,在接收返回数据时,我们需要指定一个缓冲区`buf`来存储接收到的数据,并使用`bytesRead`变量记录实际接收到的字节数。
阅读全文
相关推荐
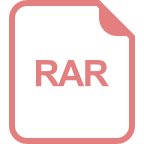

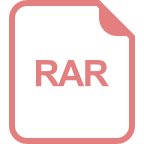













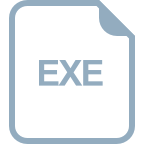