用c++语言写以下程序:双链表中一开始有个整数 0。 现在有9 次操作。每个操作会有一个操作符说明操作类型,一共有 3 类操作,每一类的操作形式如下 insert a b:在链表中整数 a 的右边插入一个整数 b delete a:在链表中删除整数 2 (不会除 0) print: 从整数 0开始向右按顺序输出所有数,整数 0 不需要输出 输入格式: 输入一个整数 9,表示有 9 次操作 接下来 9 行是每一次的操作,操作形式见题目描述 输出格式 对于每一次 print 操作,输出一行整数,中间用空格隔开,每次 print 操作后,需要换行
时间: 2024-02-04 19:02:06 浏览: 71
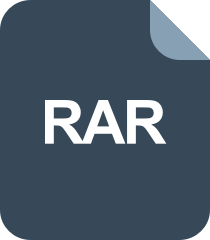
类属类链表 c++ (包括源代码、可执行文件、实验报告)

以下是用 C++ 语言实现双链表的程序:
```c++
#include <iostream>
using namespace std;
struct Node {
int val;
Node *prev, *next;
Node(int x) : val(x), prev(nullptr), next(nullptr) {}
};
class DoubleLinkedList {
private:
Node *head, *tail;
public:
DoubleLinkedList() {
head = new Node(0);
tail = head;
}
void insert(int a, int b) {
Node *cur = head;
while (cur->next && cur->val != a) {
cur = cur->next;
}
if (cur->val == a) {
Node *newNode = new Node(b);
newNode->prev = cur;
newNode->next = cur->next;
if (cur->next) {
cur->next->prev = newNode;
} else {
tail = newNode;
}
cur->next = newNode;
}
}
void remove(int a) {
Node *cur = head;
while (cur->next && cur->val != a) {
cur = cur->next;
}
if (cur->val == a && cur != head) {
cur->prev->next = cur->next;
if (cur->next) {
cur->next->prev = cur->prev;
} else {
tail = cur->prev;
}
delete cur;
}
}
void print() {
Node *cur = head->next;
while (cur) {
cout << cur->val << " ";
cur = cur->next;
}
cout << endl;
}
};
int main() {
DoubleLinkedList dll;
int n;
cin >> n;
while (n--) {
string op;
cin >> op;
if (op == "insert") {
int a, b;
cin >> a >> b;
dll.insert(a, b);
} else if (op == "delete") {
int a;
cin >> a;
dll.remove(a);
} else if (op == "print") {
dll.print();
}
}
return 0;
}
```
其中,`Node` 结构体表示链表中的每个节点,包含节点的值 `val`,以及指向前一个节点和后一个节点的指针 `prev` 和 `next`。`DoubleLinkedList` 类表示双链表,包含头节点 `head` 和尾节点 `tail`,以及插入、删除和打印链表的操作。在 `main` 函数中,根据输入的操作类型调用相应的链表操作函数。
阅读全文
相关推荐
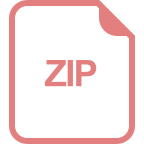
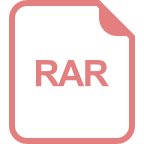















