如何从另外一台服务器上下载文件夹,java
时间: 2023-05-13 20:04:54 浏览: 467
您可以使用Java中的FTP客户端库来从另一台服务器上下载文件夹。您需要使用FTP协议连接到远程服务器,然后使用FTP客户端库中的方法下载文件夹。以下是一个简单的Java代码示例,可以从远程服务器下载文件夹:
```
import org.apache.commons.net.ftp.FTP;
import org.apache.commons.net.ftp.FTPClient;
import java.io.IOException;
public class FTPDownloadFolderExample {
public static void main(String[] args) {
String server = "remote.server.com";
int port = 21;
String user = "username";
String pass = "password";
String remoteFolderPath = "/path/to/remote/folder";
String localFolderPath = "/path/to/local/folder";
FTPClient ftpClient = new FTPClient();
try {
ftpClient.connect(server, port);
ftpClient.login(user, pass);
ftpClient.enterLocalPassiveMode();
ftpClient.setFileType(FTP.BINARY_FILE_TYPE);
downloadFolder(ftpClient, remoteFolderPath, localFolderPath);
ftpClient.logout();
ftpClient.disconnect();
} catch (IOException ex) {
ex.printStackTrace();
}
}
private static void downloadFolder(FTPClient ftpClient, String remoteFolderPath, String localFolderPath) throws IOException {
ftpClient.changeWorkingDirectory(remoteFolderPath);
String[] subDirectories = ftpClient.listNames();
for (String subDirectory : subDirectories) {
if (subDirectory.equals(".") || subDirectory.equals("..")) {
continue;
}
String remoteSubDirectoryPath = remoteFolderPath + "/" + subDirectory;
String localSubDirectoryPath = localFolderPath + "/" + subDirectory;
downloadFolder(ftpClient, remoteSubDirectoryPath, localSubDirectoryPath);
}
String[] files = ftpClient.listFiles();
for (String file : files) {
String remoteFilePath = remoteFolderPath + "/" + file;
String localFilePath = localFolderPath + "/" + file;
ftpClient.retrieveFile(remoteFilePath, new FileOutputStream(localFilePath));
}
}
}
```
在这个示例中,我们使用了Apache Commons Net库中的FTP客户端来连接到远程服务器,并使用递归函数下载了整个文件夹。请注意,您需要将`server`,`port`,`user`,`pass`,`remoteFolderPath`和`localFolderPath`替换为实际的值。
阅读全文
相关推荐
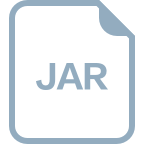













