五子棋python极大极小算法实现代码
时间: 2023-07-31 19:06:38 浏览: 54
好的,下面是一个简单的五子棋Python极大极小算法实现的代码示例:
```python
import numpy as np
# 定义棋盘大小
ROW, COL = 15, 15
# 定义棋子类型
EMPTY = 0
BLACK = 1
WHITE = 2
# 定义评估函数中的权重
SCORE_TABLE = [
[50, 500, 5000, 50000, 50000],
[10, 50, 500, 5000, 50000],
[5, 10, 50, 500, 5000],
[1, 5, 10, 50, 500],
[0, 1, 5, 10, 50]
]
# 创建棋盘
board = np.zeros((ROW, COL), dtype=int)
def is_valid_move(row, col):
# 判断落子是否在合法范围内
return row >= 0 and row < ROW and col >= 0 and col < COL and board[row][col] == EMPTY
def is_game_over(row, col):
# 判断游戏是否结束
return is_win(row, col, BLACK) or is_win(row, col, WHITE) or np.sum(board == EMPTY) == 0
def is_win(row, col, chess):
# 判断是否获胜
for i in range(5):
if (col + i < COL and board[row][col + i] == chess and
col + i - 4 >= 0 and np.all(board[row][col + i - 4:col + i + 1] == chess)):
return True
if (row + i < ROW and board[row + i][col] == chess and
row + i - 4 >= 0 and np.all(board[row + i - 4:row + i + 1, col] == chess)):
return True
if (row + i < ROW and col + i < COL and board[row + i][col + i] == chess and
row + i - 4 >= 0 and col + i - 4 >= 0 and np.all(np.diag(board[row + i - 4:row + i + 1, col + i - 4:col + i + 1]) == chess)):
return True
if (row - i >= 0 and col + i < COL and board[row - i][col + i] == chess and
row - i + 4 < ROW and col + i - 4 >= 0 and np.all(np.diag(board[row - i:row - i + 5, col + i - 4:col + i + 1]) == chess)):
return True
return False
def evaluate():
# 评估当前局势得分
score = 0
for row in range(ROW):
for col in range(COL):
if board[row][col] == BLACK:
score += SCORE_TABLE[row][col]
elif board[row][col] == WHITE:
score -= SCORE_TABLE[row][col]
return score
def minimax(depth, alpha, beta, is_maximizing_player):
if depth == 0 or is_game_over(last_move[0], last_move[1]):
return evaluate()
if is_maximizing_player:
max_eval = float('-inf')
for row in range(ROW):
for col in range(COL):
if is_valid_move(row, col):
board[row][col] = BLACK
eval = minimax(depth - 1, alpha, beta, False)
board[row][col] = EMPTY
max_eval = max(max_eval, eval)
alpha = max(alpha, eval)
if beta <= alpha:
break
return max_eval
else:
min_eval = float('inf')
for row in range(ROW):
for col in range(COL):
if is_valid_move(row, col):
board[row][col] = WHITE
eval = minimax(depth - 1, alpha, beta, True)
board[row][col] = EMPTY
min_eval = min(min_eval, eval)
beta = min(beta, eval)
if beta <= alpha:
break
return min_eval
def get_best_move():
best_eval = float('-inf')
best_move = None
for row in range(ROW):
for col in range(COL):
if is_valid_move(row, col):
board[row][col] = BLACK
eval = minimax(3, float('-inf'), float('inf'), False)
board[row][col] = EMPTY
if eval > best_eval:
best_eval = eval
best_move = (row, col)
return best_move
# 测试代码
last_move = (7, 7)
board[6][7] = WHITE
board[6][6] = BLACK
board[8][7] = WHITE
board[8][6] = BLACK
board[7][6] = WHITE
board[7][5] = BLACK
best_move = get_best_move()
print(f"Best move: {best_move}")
```
这段代码实现了一个简单的五子棋AI,使用了极大极小算法进行决策。你可以根据需要调整评估函数、搜索深度以及其他相关参数来优化算法的性能和效果。希望能帮到你!
相关推荐
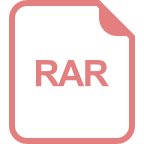














