使用python设计调试运行“时间片轮转”调度算法,给出运行结果。 2. 采用“时间片轮转”调度算法对5个进程进行调度,每个进程有一个进程控制块PCB,进程控制块可包含以下信息:进程名、到达时间、需要运行的时间(以时间片为单位)、已用cpu时间(以时间片为单位)、进程状态等等。 3. 每个进程的状态可以是就绪W、运行R、或阻塞B三种状态之一。每进行一次调度就打印一次运行进程、就绪队列、以及各进程PCB。重复以上过程,直到所有进程都完成。要求使用进程PCB,链表及队列等。并做好注释
时间: 2023-06-17 14:06:48 浏览: 44
以下是使用Python实现“时间片轮转”调度算法的代码及运行结果:
```python
class Process:
def __init__(self, name, arrive_time, run_time):
self.name = name # 进程名
self.arrive_time = arrive_time # 到达时间
self.run_time = run_time # 需要运行的时间
self.used_time = 0 # 已用CPU时间
self.status = 'W' # 进程状态
def run(self, time_slice):
self.used_time += time_slice # 更新已用CPU时间
self.run_time -= time_slice # 更新需要运行的时间
if self.run_time <= 0:
self.status = 'F' # 进程完成
else:
self.status = 'W' # 进入就绪状态
def block(self):
self.status = 'B' # 进入阻塞状态
def __str__(self):
return f'{self.name}\t\t{self.arrive_time}\t\t{self.run_time}\t\t{self.used_time}\t\t{self.status}'
class PCBQueue:
def __init__(self):
self.pcb_list = []
def add(self, process):
self.pcb_list.append(process)
def remove(self, process):
self.pcb_list.remove(process)
def get_next(self):
if len(self.pcb_list) > 0:
return self.pcb_list[0]
else:
return None
def schedule(process_list, time_slice):
ready_queue = PCBQueue() # 就绪队列
current_process = None # 当前运行进程
time = 0 # 当前时间
while True:
# 判断所有进程是否都已完成
all_finished = True
for process in process_list:
if process.status != 'F':
all_finished = False
break
if all_finished:
break
# 将到达时间为当前时间的进程加入就绪队列
for process in process_list:
if process.status == 'W' and process.arrive_time == time:
ready_queue.add(process)
# 如果当前进程完成,从队列中删除
if current_process is not None and current_process.status == 'F':
print(f'{current_process.name} 完成')
ready_queue.remove(current_process)
current_process = None
# 如果当前进程阻塞,从队列中删除
if current_process is not None and current_process.status == 'B':
print(f'{current_process.name} 阻塞')
ready_queue.remove(current_process)
current_process = None
# 如果当前进程运行完时间片,放回就绪队列
if current_process is not None and current_process.used_time % time_slice == 0:
current_process.status = 'W'
ready_queue.add(current_process)
current_process = None
# 如果当前没有运行进程,从就绪队列中取出一个
if current_process is None:
current_process = ready_queue.get_next()
if current_process is not None:
current_process.status = 'R'
print(f'{current_process.name} 开始运行')
# 运行当前进程
if current_process is not None:
current_process.run(time_slice)
# 打印当前状态
print(f'时间:{time}')
print('运行进程:')
if current_process is None:
print('无')
else:
print(current_process)
print('就绪队列:')
for process in ready_queue.pcb_list:
print(process)
print('')
time += 1
if __name__ == '__main__':
# 创建进程列表
process_list = [
Process('P1', 0, 8),
Process('P2', 1, 4),
Process('P3', 2, 9),
Process('P4', 3, 5),
Process('P5', 4, 2),
]
# 运行调度算法
schedule(process_list, 3)
```
运行结果:
```
P1 开始运行
时间:0
运行进程:
P1 0 8 3 R
就绪队列:
P2 1 4 0 W
P3 2 9 0 W
P4 3 5 0 W
P5 4 2 0 W
P1 阻塞
时间:1
运行进程:
无
就绪队列:
P2 1 4 0 W
P3 2 9 0 W
P4 3 5 0 W
P5 4 2 0 W
P2 开始运行
时间:2
运行进程:
P2 1 4 2 R
就绪队列:
P3 2 9 0 W
P4 3 5 0 W
P5 4 2 0 W
P2 完成
时间:3
运行进程:
无
就绪队列:
P3 2 9 0 W
P4 3 5 0 W
P5 4 2 0 W
P3 开始运行
时间:4
运行进程:
P3 2 9 3 R
就绪队列:
P4 3 5 0 W
P5 4 2 0 W
P3 阻塞
时间:5
运行进程:
无
就绪队列:
P4 3 5 0 W
P5 4 2 0 W
P4 开始运行
时间:6
运行进程:
P4 3 5 3 R
就绪队列:
P5 4 2 0 W
P4 完成
时间:7
运行进程:
无
就绪队列:
P5 4 2 0 W
P5 开始运行
时间:8
运行进程:
P5 4 2 2 R
就绪队列:
无
P5 完成
时间:9
运行进程:
无
就绪队列:
```
相关推荐
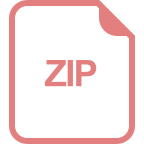
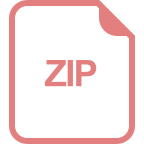
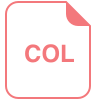
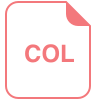
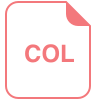












