写一段程序,使用ffmpeg解码rtsp码流并保存为文件
时间: 2023-03-12 17:28:18 浏览: 231
这是一段使用ffmpeg解码rtsp码流并保存为文件的程序:ffmpeg -i rtsp://[rtsp url] -acodec copy -vcodec copy -f mp4 [filename].mp4
相关问题
使用瑞芯微的mpp库,写段代码,要求使用ffmpeg拉rtsp流,获取到avpacket后,由mpp库调用硬件解码器解码并保存每帧画面
在使用瑞芯微的MPP库(Media Processing Platform)配合FFmpeg处理RTSP流时,首先需要安装FFmpeg,并配置它支持硬件加速解码。然后你可以按照以下步骤编写代码:
```c++
#include <mipp/mipp.h>
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/imgutils.h>
// 初始化MPP库和FFmpeg
void init_mpp_and_ffmpeg() {
mipp_init();
av_register_all();
}
// 创建FFmpeg解码上下文
AVCodecContext* create_decoder_context(const char* codec_name) {
AVCodec *codec = avcodec_find_decoder(AV_CODEC_ID_H264); // 假设H264编码
if (!codec) {
fprintf(stderr, "Failed to find decoder for H264.\n");
return nullptr;
}
AVCodecContext *ctx = avcodec_alloc_context3(codec);
if (!ctx) {
perror("Memory allocation failed.");
return nullptr;
}
if (avcodec_open2(ctx, codec, NULL) < 0) {
fprintf(stderr, "Failed to open codec.\n");
avcodec_free_context(&ctx);
return nullptr;
}
// 设置解码器特定属性(如分辨率)
ctx->width = ...; // 需要设置的实际宽度
ctx->height = ...; // 需要设置的实际高度
return ctx;
}
// 获取AVPacket并解码
void decode_frame(AVFormatContext* format_ctx, AVCodecContext* codec_ctx, AVPacket* packet, uint8_t* frame_buffer) {
while (av_read_frame(format_ctx, packet) >= 0) {
if (packet->stream_index == codec_ctx->decode_stream_index) {
int got_picture;
avcodec_send_packet(codec_ctx, packet);
avcodec_receive_frame(codec_ctx, &frame_buffer);
if (frame_buffer && avcodec_is_frame_decrypted(codec_ctx)) {
// MPP解码并处理每一帧
mipp_frame buffer_frame(frame_buffer, codec_ctx->width, codec_ctx->height);
mipp_decode(buffer_frame); // 这里使用具体的MPP函数解码
// 每帧的画面处理...
} else {
printf("Frame decoding failed.\n");
}
}
av_packet_unref(packet);
}
}
int main(int argc, char** argv) {
// 初始化
init_mpp_and_ffmpeg();
// 打开RTSP流
AVFormatContext* format_ctx = avformat_open_input(&format_ctx, "rtsp://your_rts_address", NULL, NULL);
if (!format_ctx) {
fprintf(stderr, "Failed to open RTSP stream.\n");
return -1;
}
// 查找视频流索引
int video_idx = av_find_best_stream(format_ctx, AVMEDIA_TYPE_VIDEO, -1, -1, NULL, 0);
if (video_idx < 0) {
avformat_close_input(&format_ctx);
fprintf(stderr, "No video stream found in RTSP.\n");
return -1;
}
// 获取视频解码器上下文
AVCodecContext* codec_ctx = create_decoder_context(...); // 传入实际的解码器ID
// 开始读取帧并解码
decode_frame(format_ctx, codec_ctx, &packet, ...); // 传递合适的帧缓冲区地址
// 关闭资源
avformat_close_input(&format_ctx);
avcodec_free_context(&codec_ctx);
mipp_deinit();
return 0;
}
```
请注意,上述代码是一个基础示例,实际应用中需要根据实际情况调整RTSP地址、解码器ID以及帧缓冲区管理等部分。同时,你需要确保MPP库已经包含了对指定硬件解码器的支持。
写一段c++ 使用ffmpeg库,解析rtsp流并转码到H264
以下是一段使用ffmpeg库解析rtsp流并将其转码为H264的C代码:
```
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
int main(int argc, char *argv[]) {
AVFormatContext *pFormatCtx = NULL;
int videoStream, i;
AVCodecContext *pCodecCtx = NULL;
AVCodec *pCodec = NULL;
AVFrame *pFrame = NULL;
AVPacket packet;
int frameFinished;
AVFrame *pFrameRGB = NULL;
uint8_t *buffer = NULL;
struct SwsContext *sws_ctx = NULL;
av_register_all();
// 打开rtsp流
if (avformat_open_input(&pFormatCtx, "rtsp://stream_url", NULL, NULL) != 0) {
fprintf(stderr, "Couldn't open input stream.\n");
return -1;
}
// 查找流信息
if (avformat_find_stream_info(pFormatCtx, NULL) < 0) {
fprintf(stderr, "Couldn't find stream information.\n");
return -1;
}
// 找到视频流
videoStream = -1;
for (i = 0; i < pFormatCtx->nb_streams; i++) {
if (pFormatCtx->streams[i]->codec->codec_type == AVMEDIA_TYPE_VIDEO) {
videoStream = i;
break;
}
}
if (videoStream == -1) {
fprintf(stderr, "Couldn't find video stream.\n");
return -1;
}
// 获取视频流的编解码器上下文
pCodecCtx = pFormatCtx->streams[videoStream]->codec;
// 查找解码器
pCodec = avcodec_find_decoder(pCodecCtx->codec_id);
if (pCodec == NULL) {
fprintf(stderr, "Unsupported codec!\n");
return -1;
}
// 打开解码器
if (avcodec_open2(pCodecCtx, pCodec, NULL) < 0) {
fprintf(stderr, "Could not open codec.\n");
return -1;
}
// 分配一个AVFrame并初始化
pFrame = av_frame_alloc();
pFrameRGB = av_frame_alloc();
if
阅读全文
相关推荐
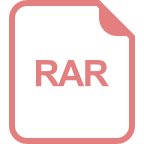
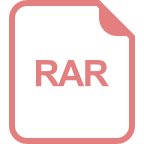
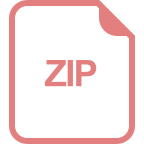
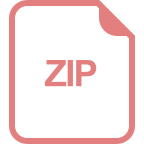
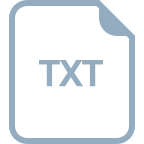
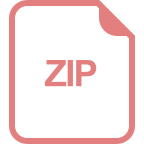
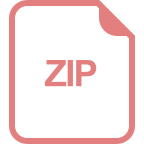
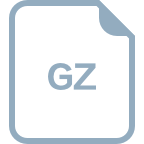
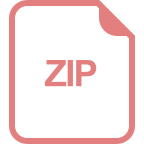
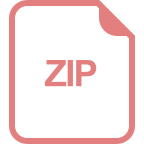





