执行该程序后,会在屏幕中央生成一个半径为200的圆,同时有一个直角扇形标识为G1,并且在圆中随机生成一个黑点。按下F键后,程序会计算黑点距离圆心的距离和圆心角度,并将扇形移动到黑点处,颜色变为红色。同时,程序会在控制台输出黑点距离圆心距离和圆心角度。
时间: 2024-05-12 13:20:13 浏览: 7
代码如下:
```python
import pygame
import math
import random
pygame.init()
# 设置屏幕
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("圆与扇形")
# 设置颜色
white = (255, 255, 255)
black = (0, 0, 0)
red = (255, 0, 0)
# 设置圆参数
circle_radius = 200
circle_center = (screen_width // 2, screen_height // 2)
# 设置扇形参数
fan_width = 200
fan_height = 200
fan_angle = 90
fan_color = white
# 随机生成黑点
black_point_radius = 5
black_point_center = (random.randint(screen_width // 2 - circle_radius, screen_width // 2 + circle_radius),
random.randint(screen_height // 2 - circle_radius, screen_height // 2 + circle_radius))
# 计算黑点距离圆心距离和圆心角度
distance = math.sqrt((black_point_center[0] - circle_center[0]) ** 2 + (black_point_center[1] - circle_center[1]) ** 2)
angle = math.atan2(black_point_center[1] - circle_center[1], black_point_center[0] - circle_center[0]) * 180 / math.pi
# 绘制圆和黑点
pygame.draw.circle(screen, white, circle_center, circle_radius, 1)
pygame.draw.circle(screen, black, black_point_center, black_point_radius)
# 绘制扇形
fan_surface = pygame.Surface((fan_width, fan_height))
fan_surface.set_colorkey((0, 0, 0))
pygame.draw.arc(fan_surface, fan_color, (0, 0, fan_width, fan_height), math.radians(180 - fan_angle), math.radians(180))
fan_surface = pygame.transform.rotate(fan_surface, angle - fan_angle // 2)
fan_rect = fan_surface.get_rect(center=black_point_center)
screen.blit(fan_surface, fan_rect)
# 显示屏幕
pygame.display.flip()
# 循环监听事件
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_f:
# 移动扇形并将颜色变为红色
fan_surface = pygame.Surface((fan_width, fan_height))
fan_surface.set_colorkey((0, 0, 0))
pygame.draw.arc(fan_surface, red, (0, 0, fan_width, fan_height), math.radians(180 - fan_angle), math.radians(180))
fan_surface = pygame.transform.rotate(fan_surface, angle - fan_angle // 2)
fan_rect = fan_surface.get_rect(center=black_point_center)
screen.blit(fan_surface, fan_rect)
pygame.display.flip()
# 输出黑点距离圆心距离和圆心角度
print("距离:", distance)
print("角度:", angle)
```
相关推荐
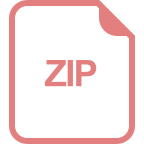














