config_toml[key] = value
时间: 2023-10-16 20:09:53 浏览: 32
这段代码是用来给 config_toml 对象中的某个键设置对应的值的。
具体来说,config_toml 是一个 JavaScript 对象,key 是一个字符串类型的键,value 是一个对应的值。通过将 value 赋值给 config_toml[key],就可以将 value 设置为 config_toml 对象中 key 对应的值。
例如,假设我们有以下的 config_toml 对象:
```
const config_toml = {
name: "my-app",
port: 3000,
database: {
host: "localhost",
port: 27017,
name: "my-database"
}
}
```
如果我们想要将 config_toml 对象中的 port 值修改为 4000,可以使用以下代码:
```
config_toml['port'] = 4000;
```
或者:
```
config_toml.port = 4000;
```
这将把 port 的值从 3000 修改为 4000。
相关问题
修改toml文件config_toml[key] = value
要修改一个 toml 文件中的某个键对应的值,你需要先读取该文件,然后修改相应的键值,最后再将修改后的结果写回到文件中。
以下是一个使用 `toml` 模块读取和写入 toml 文件的示例:
```
const fs = require('fs');
const toml = require('toml');
// 读取 toml 文件
const fileContent = fs.readFileSync('config.toml', 'utf8');
// 解析 toml 文件
const config_toml = toml.parse(fileContent);
// 修改 toml 文件中的键值
config_toml['port'] = 4000;
// 转换回 toml 格式的字符串
const updatedContent = toml.stringify(config_toml);
// 将修改后的内容写回到文件
fs.writeFileSync('config.toml', updatedContent, 'utf8');
```
在上面的示例中,我们首先使用 `fs` 模块读取 `config.toml` 文件的内容,然后使用 `toml` 模块解析该文件内容并将其转换成一个 JavaScript 对象。接着,我们修改了该对象中的 `port` 键对应的值,并使用 `toml` 模块将修改后的对象转换回 toml 格式的字符串。最后,我们使用 `fs` 模块将修改后的字符串写回到 `config.toml` 文件中。
需要注意的是,该示例中的代码仅演示了如何修改 toml 文件中的一个键值,如果 toml 文件中包含多个键值需要修改,你需要相应地修改代码。
fs.writeFileSync('config_toml.toml', toml.stringify(config_toml))
`.writeFileSync()` 是 Node.js 的一个文件系统模块中的方法,用于将数据写入到文件中。在这个例子中,我们使用 `fs.writeFileSync()` 方法将一个 JavaScript 对象 `config_toml` 转换为 toml 格式的字符串,并将该字符串写入到名为 `config_toml.toml` 的文件中。以下是一个例子:
```javascript
const fs = require('fs');
const toml = require('toml');
const config_toml = {
title: 'Example',
author: {
name: 'John Smith',
email: 'john@example.com'
},
tags: ['javascript', 'node.js', 'toml']
};
fs.writeFileSync('config_toml.toml', toml.stringify(config_toml));
```
在这个例子中,我们首先导入了 `fs` 和 `toml` 模块,并定义了一个 JavaScript 对象 `config_toml`,该对象包含了一些数据。然后,我们调用 `toml.stringify()` 方法,并将 `config_toml` 对象作为参数传递给该方法,将其转换为 toml 格式的字符串。最后,我们使用 `fs.writeFileSync()` 方法,将 toml 格式的字符串写入到名为 `config_toml.toml` 的文件中。
需要注意的是,如果目标文件已经存在,`fs.writeFileSync()` 方法会覆盖原有的文件内容。如果需要追加数据到文件末尾,可以使用 `fs.appendFileSync()` 方法。在写入文件时,需要确保目标文件的路径存在,并且具有写入权限。
另外,如果在写入文件时发生了错误,`fs.writeFileSync()` 方法会抛出异常,可以使用 `try...catch` 语句来处理这些异常。
相关推荐
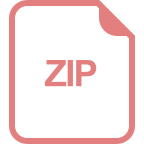












