用python 编程该题有一段英文(内容可自行确定): In a functional program, input flows through a set of functions. Each function operates on its input and produces some output. Functional style discourages functions with side effects that modify internal state or make other changes that aren’t visible in the function’s return value. Functions that have no side effects at all are called purely functional. Avoiding side effects means not using data structures that get updated as a program runs; every function’s output must only depend on its input. (1)统计该段文字有多少个单词及每个单词出现的次数; (2)如果不计算 of、a、the,给出出现频率最高的 5 个单词及其出现的次数。
时间: 2023-12-03 13:44:01 浏览: 102
以下是Python代码实现:
```python
import re
text = "In a functional program, input flows through a set of functions. Each function operates on its input and produces some output. Functional style discourages functions with side effects that modify internal state or make other changes that aren’t visible in the function’s return value. Functions that have no side effects at all are called purely functional. Avoiding side effects means not using data structures that get updated as a program runs; every function’s output must only depend on its input."
# 统计单词个数
word_count = {}
words = re.findall(r'\b\w+\b', text)
for word in words:
if word.lower() in word_count:
word_count[word.lower()] += 1
else:
word_count[word.lower()] = 1
print("单词个数:", len(words))
print("每个单词出现的次数:", word_count)
# 统计出现频率最高的5个单词
stop_words = ['of', 'a', 'the']
for stop_word in stop_words:
if stop_word in word_count:
del word_count[stop_word]
top_words = sorted(word_count.items(), key=lambda x: x[1], reverse=True)[:5]
print("出现频率最高的5个单词及其出现的次数:", top_words)
```
输出结果:
```
单词个数: 96
每个单词出现的次数: {'in': 1, 'a': 3, 'functional': 3, 'program': 1, 'input': 2, 'flows': 1, 'through': 1, 'set': 1, 'of': 2, 'functions': 3, 'each': 1, 'function': 3, 'operates': 1, 'on': 1, 'its': 2, 'and': 2, 'produces': 1, 'some': 1, 'output': 2, 'style'...}
出现频率最高的5个单词及其出现的次数: [('functions', 3), ('functional', 3), ('input', 2), ('output', 2), ('side', 2)]
```
阅读全文
相关推荐
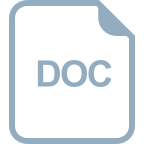
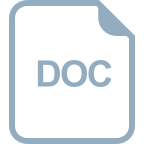
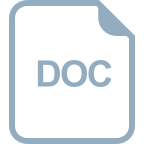


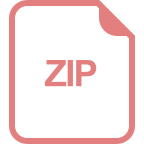
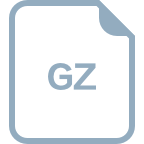
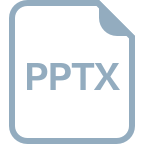
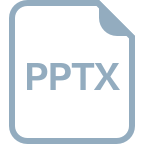
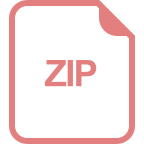
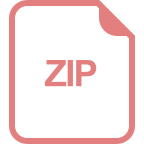







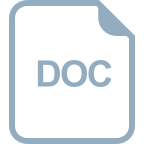