precision and recall 实现
时间: 2024-02-03 15:01:45 浏览: 46
Precision (精确率) 和 Recall (召回率) 是机器学习中的两个重要指标,用于评估分类模型的性能。精确率指预测为正的样本中真正为正的样本占比,召回率指真正为正的样本中被正确地预测为正的样本占比。以二分类问题为例,精确率和召回率的计算公式如下:
Precision = TP/(TP+FP)
Recall = TP/(TP+FN)
其中,TP 表示真正为正的样本预测为正,FP 表示真正为负的样本预测为正,FN 表示真正为正的样本预测为负。通常情况下,精确率和召回率是相互矛盾的,提高精确率会降低召回率,反之亦然。因此,常常使用 F1-Score 来综合评价模型的性能,其计算公式如下:
F1-Score = (2 * Precision * Recall) / (Precision + Recall)
相关问题
precision-recall曲线python代码实现
好的,以下是一个简单的 precision-recall 曲线的 Python 代码实现:
```python
import numpy as np
import matplotlib.pyplot as plt
# 生成模拟数据
predictions = np.random.rand(100)
labels = np.random.randint(2, size=100)
# 计算精确度和召回率
tp = np.sum(np.logical_and(predictions == 1, labels == 1))
fp = np.sum(np.logical_and(predictions == 1, labels == 0))
tn = np.sum(np.logical_and(predictions == 0, labels == 0))
fn = np.sum(np.logical_and(predictions == 0, labels == 1))
precision = tp / (tp + fp)
recall = tp / (tp + fn)
# 计算不同阈值下的精确度和召回率,并绘制曲线
thresholds = np.arange(0, 1.01, 0.01)
precisions = []
recalls = []
for t in thresholds:
pred = np.where(predictions >= t, 1, 0)
tp = np.sum(np.logical_and(pred == 1, labels == 1))
fp = np.sum(np.logical_and(pred == 1, labels == 0))
fn = np.sum(np.logical_and(pred == 0, labels == 1))
p = tp / (tp + fp) if tp + fp != 0 else 0
r = tp / (tp + fn) if tp + fn != 0 else 0
precisions.append(p)
recalls.append(r)
plt.plot(recalls, precisions)
plt.xlabel("Recall")
plt.ylabel("Precision")
plt.title("Precision-Recall Curve")
plt.show()
```
在这个例子中,我们生成了一些随机的二元标签和预测值,然后计算了一个阈值下的精确度和召回率,最后计算了不同阈值下的精确度和召回率,并绘制了 precision-recall 曲线。
用python实现recall和precision
可以使用以下代码实现recall和precision:
```python
def recall(true_positives, false_negatives):
"""
计算召回率
"""
return true_positives / (true_positives + false_negatives)
def precision(true_positives, false_positives):
"""
计算精确度
"""
return true_positives / (true_positives + false_positives)
```
其中,`true_positives` 表示真正例的数量,`false_positives` 表示假正例的数量,`false_negatives` 表示假反例的数量。
例如,对于一个二分类模型,可以使用以下代码计算其recall和precision:
```python
# 模型预测结果
y_pred = [1, 0, 1, 1, 0, 1, 0, 0, 1, 1]
# 真实标签
y_true = [1, 0, 1, 0, 1, 0, 1, 0, 1, 0]
# 计算真正例、假正例和假反例的数量
true_positives = sum([1 for i in range(len(y_true)) if y_true[i] == 1 and y_pred[i] == 1])
false_positives = sum([1 for i in range(len(y_true)) if y_true[i] == 0 and y_pred[i] == 1])
false_negatives = sum([1 for i in range(len(y_true)) if y_true[i] == 1 and y_pred[i] == 0])
# 计算召回率和精确度
recall_score = recall(true_positives, false_negatives)
precision_score = precision(true_positives, false_positives)
print("Recall: ", recall_score)
print("Precision: ", precision_score)
```
输出结果如下:
```
Recall: 0.6
Precision: 0.6666666666666666
```
这表示模型的召回率为 0.6,精确度为 0.67。
相关推荐
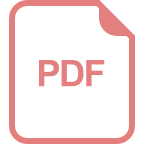
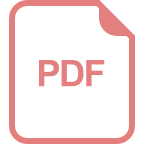












