如何在一组无序的字符串中查找特定字符并获取其出现的所有位置?
时间: 2024-12-21 10:26:29 浏览: 5
在一组无序的字符串列表中查找特定字符并获取其所有出现的位置,可以按照以下步骤操作:
1. 首先,遍历整个字符串列表。对于每个字符串,你可以使用循环结构逐个检查每一个字符。
2. 使用条件判断,比如 `if` 语句,检查当前字符是否等于你要查找的目标字符。
3. 如果匹配,则记录下当前字符串中该字符的位置。这通常通过索引来完成,例如数组下标或字符串的 `index()` 或 `find()` 函数。
4. 当找到目标字符后,在列表中存储其位置,可以是一个列表,用于存放所有出现的位置,或者直接将位置添加到某个数据结构如集合(Set)中,避免重复。
5. 继续遍历直到检查完整个字符串。如果在所有字符串中都没有找到,那么返回的结果就是空列表或表示未找到的标识。
以下是一个简单的 Python 示例:
```python
def find_positions(strings, target_char):
positions = [] # 存储结果的列表
for i, string in enumerate(strings):
index = string.find(target_char)
while index != -1: # -1 表示未找到
positions.append(index) # 添加位置
index = string.find(target_char, index + 1) # 继续搜索下一个位置
return positions
strings = ["hello", "world", "hello again"]
target_char = "l"
positions = find_positions(strings, target_char)
print(f"字符 '{target_char}' 出现的位置: {positions}")
```
阅读全文
相关推荐
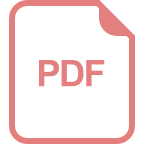
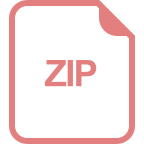
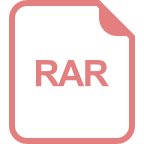
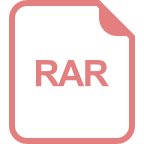
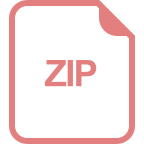
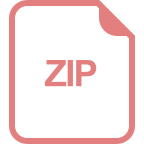
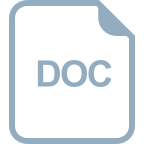
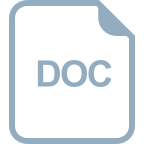
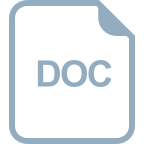
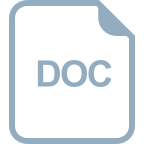
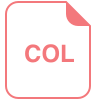
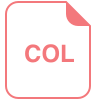
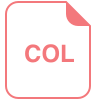
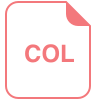
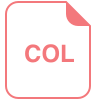
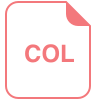
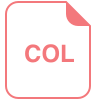
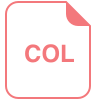
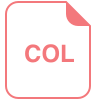
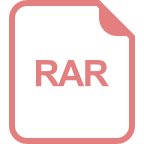