利用vue3 element-plus写一个漂亮的带图片的添加页面
时间: 2024-02-01 10:15:05 浏览: 114
好的,下面是一个简单的示例代码。首先,你需要安装 Vue3 和 Element Plus,并在你的项目中导入它们。
```html
<template>
<div class="add-page">
<el-card class="add-card">
<h3 class="add-title">添加商品</h3>
<el-form ref="addForm" :model="form" label-width="80px">
<el-form-item label="商品名称">
<el-input v-model="form.name"></el-input>
</el-form-item>
<el-form-item label="商品图片">
<el-upload
class="avatar-uploader"
action="/api/upload"
:show-file-list="false"
:on-success="handleUploadSuccess"
:before-upload="beforeUpload"
>
<img v-if="imageUrl" :src="imageUrl" class="avatar">
<i v-else class="el-icon-plus avatar-uploader-icon"></i>
</el-upload>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="submitForm">添加</el-button>
<el-button @click="resetForm">重置</el-button>
</el-form-item>
</el-form>
</el-card>
</div>
</template>
<script>
import { ref } from 'vue';
import { ElCard, ElForm, ElFormItem, ElInput, ElUpload, ElButton } from 'element-plus';
export default {
components: { ElCard, ElForm, ElFormItem, ElInput, ElUpload, ElButton },
setup() {
const form = ref({
name: '',
imageUrl: '',
});
const imageUrl = ref('');
const handleUploadSuccess = (response, file, fileList) => {
this.imageUrl = URL.createObjectURL(file.raw);
this.form.imageUrl = response.url;
};
const beforeUpload = (file) => {
const isJpgOrPng = file.type === 'image/jpeg' || file.type === 'image/png';
if (!isJpgOrPng) {
this.$message.error('只能上传 JPG/PNG 格式的文件');
return false;
}
const isLt2M = file.size / 1024 / 1024 < 2;
if (!isLt2M) {
this.$message.error('文件大小不能超过 2MB');
return false;
}
return true;
};
const submitForm = () => {
this.$refs.addForm.validate((valid) => {
if (valid) {
// 提交表单
} else {
console.log('error submit!!');
return false;
}
});
};
const resetForm = () => {
this.$refs.addForm.resetFields();
this.imageUrl = '';
this.form.imageUrl = '';
};
return {
form,
imageUrl,
handleUploadSuccess,
beforeUpload,
submitForm,
resetForm,
};
},
};
</script>
<style scoped>
.add-page {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.add-card {
width: 600px;
}
.add-title {
text-align: center;
font-size: 24px;
margin-bottom: 20px;
}
.avatar-uploader {
display: flex;
justify-content: center;
align-items: center;
width: 120px;
height: 120px;
border: 1px solid #ccc;
border-radius: 50%;
overflow: hidden;
margin: 0 auto;
}
.avatar-uploader-icon {
font-size: 28px;
color: #999;
}
.avatar {
width: 100%;
height: 100%;
object-fit: cover;
}
</style>
```
这是一个简单的添加商品页面,包含一个表单 和 上传图片组件。你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
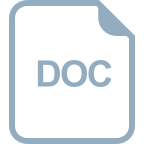
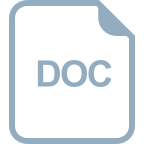
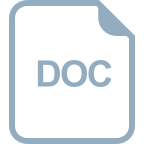
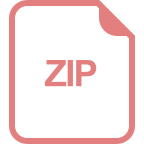

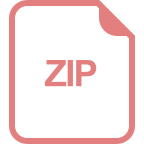
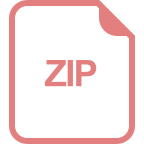











