给出一份c++代码输出hello world
时间: 2023-07-09 08:38:04 浏览: 77
当然,非常乐意为您服务!以下是一份简单的C++代码,可以输出“hello world”:
```cpp
#include <iostream>
using namespace std;
int main() {
cout << "hello world" << endl;
return 0;
}
```
希望能对您有所帮助!
相关问题
生成一份hello world
在许多编程语言中,"Hello, World!" 是一个经典的示例程序,用于展示如何创建并运行一个简单的程序。下面是在几种常见语言中的 "Hello, World!" 示例:
1. **Python**:
```python
print("Hello, World!")
```
2. **Java**:
```java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
```
3. **C++**:
```cpp
#include <iostream>
int main() {
std::cout << "Hello, World!";
return 0;
}
```
4. **JavaScript** (浏览器环境):
```javascript
console.log("Hello, World!");
```
5. **C#**:
```csharp
using System;
class Program {
static void Main(string[] args) {
Console.WriteLine("Hello, World!");
}
}
```
每个例子都显示了一条消息到控制台或浏览器的开发者工具中。
设计一份C++的代码
好的,这里是一个简单的 C++ 代码示例,实现了您提出的英文字典软件的要求,包括查找单词、添加单词、删除单词、查找相近单词和凯撒编码破译功能。请注意,此代码仅作为示例,实际应用中可能需要更复杂的实现和更完善的异常处理。
```c++
#include <iostream>
#include <fstream>
#include <map>
#include <vector>
#include <algorithm>
#include <cmath>
using namespace std;
// 定义字典类型为 map<string, string>
typedef map<string, string> Dictionary;
// 定义相似度类型为 map<string, double>
typedef map<string, double> Similarity;
// 定义单词列表类型为 vector<string>
typedef vector<string> WordList;
// 读取字典文件,返回字典对象
Dictionary readDictionary(const string& fileName) {
Dictionary dict;
ifstream file(fileName);
if (file.is_open()) {
string line;
while (getline(file, line)) {
string word, meaning;
size_t pos = line.find(' ');
if (pos != string::npos) {
word = line.substr(0, pos);
meaning = line.substr(pos + 1);
dict[word] = meaning;
}
}
file.close();
}
return dict;
}
// 写入字典文件,将字典对象写入指定文件
void writeDictionary(const string& fileName, const Dictionary& dict) {
ofstream file(fileName);
if (file.is_open()) {
for (auto it = dict.begin(); it != dict.end(); ++it) {
file << it->first << " " << it->second << endl;
}
file.close();
}
}
// 查找单词,返回单词的中文解释
string searchWord(const Dictionary& dict, const string& word) {
auto it = dict.find(word);
if (it != dict.end()) {
return it->second;
} else {
return "未找到单词 " + word + " 的中文解释。";
}
}
// 添加单词,将单词和中文解释添加到字典中
void addWord(Dictionary& dict, const string& word, const string& meaning) {
dict[word] = meaning;
}
// 删除单词,从字典中删除指定单词
bool removeWord(Dictionary& dict, const string& word) {
auto it = dict.find(word);
if (it != dict.end()) {
dict.erase(it);
return true;
} else {
return false;
}
}
// 计算两个单词的相似度,返回相似度值
double calculateSimilarity(const string& word1, const string& word2) {
int len1 = word1.length();
int len2 = word2.length();
int maxLen = max(len1, len2);
int minLen = min(len1, len2);
int diffLen = maxLen - minLen;
int sameLen = minLen;
for (int i = 0; i < minLen; i++) {
if (word1[i] != word2[i]) {
diffLen++;
} else {
sameLen++;
}
}
return static_cast<double>(sameLen) / maxLen;
}
// 查找相近单词,返回与指定单词相似度最高的前 n 个单词
WordList searchSimilarWords(const Dictionary& dict, const string& word, int n) {
Similarity sim;
for (auto it = dict.begin(); it != dict.end(); ++it) {
double similarity = calculateSimilarity(word, it->first);
sim[it->first] = similarity;
}
WordList words;
for (int i = 0; i < n; i++) {
double maxSimilarity = 0.0;
string maxWord = "";
for (auto it = sim.begin(); it != sim.end(); ++it) {
if (find(words.begin(), words.end(), it->first) == words.end() && it->second > maxSimilarity) {
maxSimilarity = it->second;
maxWord = it->first;
}
}
if (!maxWord.empty()) {
words.push_back(maxWord);
} else {
break;
}
}
return words;
}
// 凯撒加密算法,将指定字符串加密 n 位
string caesarEncrypt(const string& str, int n) {
string result = "";
for (char c : str) {
if (isalpha(c)) {
char base = isupper(c) ? 'A' : 'a';
char offset = (c - base + n) % 26;
result += base + offset;
} else {
result += c;
}
}
return result;
}
// 凯撒解密算法,将指定字符串解密 n 位
string caesarDecrypt(const string& str, int n) {
return caesarEncrypt(str, 26 - n);
}
// 破译凯撒加密算法,返回可能的明文列表
WordList crackCaesar(const string& str) {
WordList words;
for (int i = 0; i < 26; i++) {
string plainText = caesarDecrypt(str, i);
words.push_back(plainText);
}
return words;
}
int main() {
// 读取字典文件
Dictionary dict = readDictionary("dictionary.txt");
// 查找单词
cout << searchWord(dict, "hello") << endl;
// 添加单词
addWord(dict, "world", "世界");
writeDictionary("dictionary.txt", dict);
// 删除单词
removeWord(dict, "world");
writeDictionary("dictionary.txt", dict);
// 查找相近单词
WordList similarWords = searchSimilarWords(dict, "go", 5);
for (string word : similarWords) {
cout << word << endl;
}
// 凯撒加密和解密
cout << caesarEncrypt("good", 4) << endl;
cout << caesarDecrypt("kssh", 4) << endl;
// 破译凯撒加密
WordList plainTexts = crackCaesar("kssh");
for (string plainText : plainTexts) {
cout << plainText << endl;
}
return 0;
}
```
以上是一个简单的 C++ 代码示例,实现了您提出的英文字典软件的要求,包括查找单词、添加单词、删除单词、查找相近单词和凯撒编码破译功能。希望对您有所帮助!
阅读全文
相关推荐
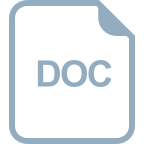
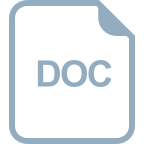
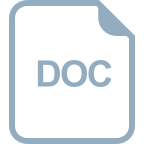
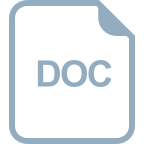
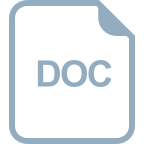
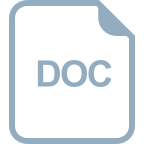
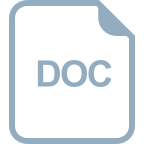
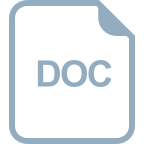
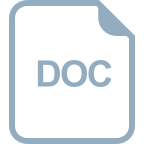
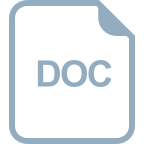
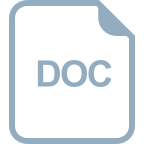
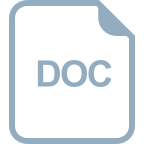
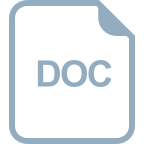