Java 使用MySQL建立一个名为bank的数据库,在bank数据库中创建card1和card2 表,card1和card2表的字段如下(两者相同): number(char),name(char), amount(float),其中number字段为主键。 程序进行两个操作,一是将card1表中某记录的amount字段的值减去100,二 是将card2表中某记录的amount字段的值增加100,必须保证这两个操作要么 成功,要么失败。
时间: 2024-02-10 16:14:42 浏览: 61
好的,这是一个关于Java和MySQL的问题。下面是我给出的答案:
首先,我们需要在MySQL中创建一个名为bank的数据库,并在该数据库中创建card1和card2表,如下所示:
```
CREATE DATABASE bank;
USE bank;
CREATE TABLE card1 (
number CHAR(20) PRIMARY KEY,
name CHAR(50),
amount FLOAT
);
CREATE TABLE card2 (
number CHAR(20) PRIMARY KEY,
name CHAR(50),
amount FLOAT
);
```
接下来,我们可以使用Java程序对这两个表进行操作。在Java中,我们可以使用JDBC来连接MySQL数据库并执行SQL查询。下面是一个Java程序,用于将card1表中某记录的amount字段的值减去100,并将card2表中某记录的amount字段的值增加100:
```
import java.sql.*;
public class BankTransaction {
public static void main(String[] args) {
Connection conn = null;
PreparedStatement stmt1 = null;
PreparedStatement stmt2 = null;
ResultSet rs1 = null;
ResultSet rs2 = null;
try {
// Connect to the database
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost/bank", "username", "password");
// Start a transaction
conn.setAutoCommit(false);
// Decrease amount in card1 table
stmt1 = conn.prepareStatement("SELECT amount FROM card1 WHERE number = ?");
stmt1.setString(1, "1234567890"); // Replace with the actual number
rs1 = stmt1.executeQuery();
if (rs1.next()) {
float amount = rs1.getFloat("amount");
if (amount >= 100) {
stmt1 = conn.prepareStatement("UPDATE card1 SET amount = amount - 100 WHERE number = ?");
stmt1.setString(1, "1234567890"); // Replace with the actual number
stmt1.executeUpdate();
} else {
throw new Exception("Insufficient balance");
}
} else {
throw new Exception("Record not found");
}
// Increase amount in card2 table
stmt2 = conn.prepareStatement("SELECT amount FROM card2 WHERE number = ?");
stmt2.setString(1, "0987654321"); // Replace with the actual number
rs2 = stmt2.executeQuery();
if (rs2.next()) {
stmt2 = conn.prepareStatement("UPDATE card2 SET amount = amount + 100 WHERE number = ?");
stmt2.setString(1, "0987654321"); // Replace with the actual number
stmt2.executeUpdate();
} else {
throw new Exception("Record not found");
}
// Commit the transaction
conn.commit();
System.out.println("Transaction completed successfully");
} catch (Exception e) {
try {
// Rollback the transaction if any error occurs
if (conn != null) {
conn.rollback();
}
} catch (SQLException ex) {
ex.printStackTrace();
}
e.printStackTrace();
} finally {
try {
// Close all the resources
if (rs1 != null) rs1.close();
if (rs2 != null) rs2.close();
if (stmt1 != null) stmt1.close();
if (stmt2 != null) stmt2.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
在上面的代码中,我们首先连接到MySQL数据库,并设置自动提交为false,以启动一个事务。然后,我们使用PreparedStatement对象分别查询card1和card2表中的记录,并根据查询结果更新相应的记录。如果任何一个操作失败,我们将回滚整个事务并抛出异常。如果两个操作都成功,我们将提交事务并输出一条成功消息。
请注意,上面的代码中的用户名和密码应替换为实际的值。此外,需要将卡号(number)替换为实际的值,以便正确地查询和更新记录。
相关推荐
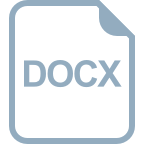
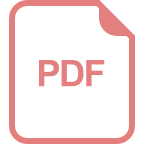
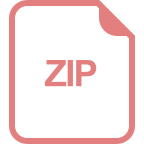















