生成十个随机目标点并输出位置坐标,再用麻雀搜索算法进行路径规划,要求每个目标点只被访问一次,最后输出最短路径 Python代码
时间: 2024-03-22 16:41:27 浏览: 14
好的,我理解了你的问题。根据你的需求,我可以给出以下 Python 代码来实现:
```python
import random
import math
# 生成随机目标点
targets = []
for i in range(10):
x = random.uniform(0, 100)
y = random.uniform(0, 100)
targets.append((x, y))
print(f"目标点{i + 1}: ({x:.2f}, {y:.2f})")
# 计算两点之间的距离
def distance(point1, point2):
x1, y1 = point1
x2, y2 = point2
return math.sqrt((x1 - x2) ** 2 + (y1 - y2) ** 2)
# 麻雀搜索算法
def sparrow_search(targets):
# 起点为坐标原点
current_point = (0, 0)
# 已访问过的目标点
visited_targets = []
# 计算起点到每个目标点的距离
distances = [distance(current_point, target) for target in targets]
# 搜索路径
path = []
# 遍历所有目标点
while len(visited_targets) < len(targets):
# 找到距离当前点最近的未访问目标点
min_distance = float('inf')
next_point = None
for i in range(len(targets)):
if i not in visited_targets and distances[i] < min_distance:
min_distance = distances[i]
next_point = targets[i]
# 更新当前点和已访问目标点列表
current_point = next_point
visited_targets.append(targets.index(current_point))
# 更新距离列表
for i in range(len(targets)):
if i not in visited_targets:
distances[i] = min(distances[i], distance(current_point, targets[i]))
# 将当前点加入路径
path.append(current_point)
# 添加回原点的路径
path.append((0, 0))
# 计算总路径长度
total_distance = sum([distance(path[i], path[i+1]) for i in range(len(path)-1)])
return path, total_distance
# 运行搜索算法并输出结果
path, total_distance = sparrow_search(targets)
print(f"最短路径为:{path}")
print(f"路径长度为:{total_distance:.2f}")
```
代码中,首先生成了十个随机目标点,并输出它们的坐标。然后定义了一个计算两点之间距离的函数 `distance`,以及一个麻雀搜索算法 `sparrow_search`。在算法中,起点默认为坐标原点,已访问的目标点列表 `visited_targets` 初始为空,每次从未访问的目标点中选择距离当前点最近的点作为下一个目标点,并更新距离列表 `distances`。最终得到的路径是一个列表,包括了所有的目标点和回到原点的路径,总路径长度通过计算每两个相邻点之间的距离求和得到。最后输出最短路径和路径长度。
希望这段代码可以帮助到你,如果还有什么疑问可以随时问我。
相关推荐
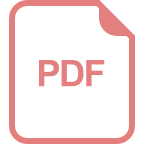














