#include<iostream> using namespace std; class student; class teacher { public: int a = 1; student *p; teacher(int a); ~teacher(); }; teacher::teacher(int a) { cout << "teacher构造函数调用" << endl; this->a = a; p = new student; } teacher::~teacher() { cout << "teacher析构函数调用" << endl; delete p; } class student { public: //友元函数可以访问类中的公有和私有成员,不可以访问保护成员 friend void func2(student &s); friend class teacher; int a = 10; student(int x); ~student(); void func3(); private: int b = 20; }; student::student(int x) :a(x) { cout << "student 构造函数调用1" << endl; } student::~student() { cout << "student 析构函数调用!" << endl; } void student::func3() { cout << b << endl; } void func1() { student s1(100); cout << s1.a << endl; s1.func3(); func2(s1); cout << s1.a << endl; } //友元函数,全局函数做友元 //在形参中加入const防止传入的参数被改变,不加const则可以改变 void func2( student &s) { //传入普通类对象不可以改变类中的值,只能改变形参类的值 /s.a = 123; cout<<s.a << endl; cout << s.b << endl;/ ////传入指针可以改变类的值 /s->a = 1000; s->b = 123; cout << s->a << endl; cout << s->b << endl;/ ///传入引用也可以改变类中的值 //s.a = 12345; //s.b = 123456; cout << s.a << endl; cout << s.b << endl; } //类做友元 void func3() { student s1(10); cout << s1.a << endl; teacher t1(100); cout << t1.a << endl; cout << t1.p->a<< endl; } int main() { //成员函数做友元 //func1(); //类做友元 func3(); system("pause"); return 0; }
时间: 2023-10-30 10:57:39 浏览: 49
这段代码是一个简单的 C++ 程序,它定义了两个类:`teacher` 和 `student`。其中 `teacher` 类有一个指向 `student` 类对象的指针成员 `p`,并且定义了构造函数和析构函数;`student` 类定义了构造函数、析构函数和一个成员函数 `func3()`,并且有一个私有成员变量 `b`。此外,程序还定义了若干个全局函数,包括一个友元函数 `func2()`,它可以访问 `student` 类的私有成员变量 `b`。最后,在 `main()` 函数中,程序演示了如何使用这些类和函数。
相关问题
#include<iostream> using namespace std; class student; class teacher { public : student *p; teacher(int x); int A; ~teacher(); }; teacher::teacher(int x) { A = x; p = new student(8); } teacher::~teacher() { delete p; } class student { friend class teacher; public: int a = 10; student(int x); }; student::student(int x) { a = x; } void func() { student s(100); cout << s.a << endl; teacher t(1); cout << t.A << endl; cout << t.p->a << endl; } int main() { func(); system("pause"); return 0; }这个代码问题出现在哪,该怎么解决
这段代码存在一个循环包含的问题,即 `class teacher` 中的 `student *p` 指针指向了 `class student`,而 `class student` 中又要使用 `class teacher`,这就导致了编译器无法处理两个类的定义顺序。要解决这个问题,可以将 `class student` 的定义放在 `class teacher` 的前面,或者使用前置声明来解决。修正后的代码如下:
```
#include<iostream>
using namespace std;
class student;
class teacher {
public:
student *p;
teacher(int x);
int A;
~teacher();
};
class student {
friend class teacher;
public:
int a = 10;
student(int x);
};
student::student(int x) {
a = x;
}
teacher::teacher(int x) {
A = x;
p = new student(8);
}
teacher::~teacher() {
delete p;
}
void func() {
student s(100);
cout << s.a << endl;
teacher t(1);
cout << t.A << endl;
cout << t.p->a << endl;
}
int main() {
func();
system("pause");
return 0;
}
```
#include<iostream> using namespace std; class student; class teacher { public : student *p; //student p; teacher(int x); int A; ~teacher(); }; class student { friend class teacher; public: int a = 10; student(int x); private: int b = 100; }; teacher::teacher(int x) { A = x; p = new student(8); } teacher::~teacher() { delete p; } student::student(int x) { a = x; } void func() { student s(100); cout << s.a << endl; teacher t(1); cout << t.A << endl; cout << t.p->a << endl; cout << t.p->b << endl; } int main() { func(); system("pause"); return 0; }
这段代码定义了两个类 `student` 和 `teacher`,`teacher` 类中包含一个指向 `student` 类对象的指针,同时 `student` 类也声明了 `teacher` 类为其友元类。在 `main` 函数中,先定义一个 `student` 对象 `s`,输出 `s.a` 的值,然后定义一个 `teacher` 对象 `t`,输出 `t.A` 的值、`t.p->a` 的值以及 `t.p->b` 的值。
运行这段代码会输出以下结果:
```
100
1
8
100
```
这说明在 `teacher` 类中,通过 `p` 指针成功访问了 `student` 类的私有成员 `a` 和 `b`,这是因为 `student` 类将 `teacher` 类声明为其友元类,使得 `teacher` 类可以访问 `student` 类的私有成员。需要注意的是,这里使用了动态内存分配,需要在 `teacher` 类的析构函数中释放内存。
相关推荐
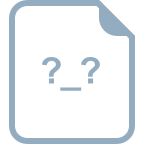














