Python随机圆
时间: 2023-08-14 08:15:14 浏览: 193
要生成随机圆,可以使用Python中的turtle库。下面是一个简单的示例代码:
```python
import turtle
import random
# 创建画布和画笔
canvas = turtle.Screen()
pen = turtle.Turtle()
# 设置画笔的颜色和形状
pen.color("blue")
pen.shape("circle")
pen.penup()
# 生成随机圆的位置和半径
x = random.randint(-200, 200)
y = random.randint(-200, 200)
r = random.randint(50, 100)
# 移动画笔到圆的位置
pen.goto(x, y)
# 画圆
pen.pendown()
pen.circle(r)
# 隐藏画笔
pen.hideturtle()
# 显示画布
canvas.mainloop()
```
运行上述代码,将会在画布中生成一个随机位置和大小的圆形。你可以多运行几次代码,生成不同的随机圆。
相关问题
python随机绘制圆
Python随机绘制圆可以使用Pygame库中的pygame.draw.circle()函数。该函数需要传入屏幕对象、颜色、圆心坐标、半径和线宽等参数。可以使用random库生成随机的圆心坐标和半径,从而实现随机绘制圆的效果。
在绘制圆的过程中,可以使用数学库中的math.hypot()函数计算两个圆心之间的距离,从而判断是否与其他圆重叠。如果重叠,则可以设置一个变量shouldprint为false,不进行绘制。
代码示例:
```
import pygame
import random
import math
pygame.init()
# 设置屏幕大小和标题
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Random Circles")
# 定义颜色
red = (255, 0, 0)
# 定义圆类
class Circle:
def __init__(self):
self.x = random.randint(0, 800)
self.y = random.randint(0, 600)
self.r = random.randint(10, 50)
self.shouldprint = True
def check_collision(self, c):
for i in range(len(c)):
if c[i] != self:
dist = int(math.hypot(c[i].x - self.x, c[i].y - self.y))
if dist < c[i].r + self.r:
self.shouldprint = False
break
def draw(self):
if self.shouldprint:
pygame.draw.circle(screen, red, (self.x, self.y), self.r, 1)
# 创建圆列表
circles = []
for i in range(50):
c = Circle()
c.check_collision(circles)
circles.append(c)
# 游戏循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 绘制圆
for c in circles:
c.draw()
# 更新屏幕
pygame.display.update()
# 退出游戏
pygame.quit()
```
python随机多个绘制不重合的圆
在Python中,你可以使用matplotlib库来创建不重叠的随机圆形图。以下是一个简单的示例,展示了如何使用`plt.scatter()`函数结合一些随机生成的坐标来绘制随机分布的圆:
```python
import matplotlib.pyplot as plt
import numpy as np
# 设置画布大小和背景颜色
fig, ax = plt.subplots(figsize=(8, 8), facecolor='lightgray')
# 随机生成圆心位置和半径
n_circles = 10 # 圆的数量
circle_centers = np.random.rand(n_circles, 2) * 5 + [2, 2] # x, y坐标范围在(2,7)×(2,7)
circle_radii = np.random.uniform(0.5, 2, n_circles)
# 绘制不重叠的圆
for center, radius in zip(circle_centers, circle_radii):
ax.add_patch(plt.Circle(center, radius, fill=False)) # 填充为False表示只是边框
# 设置标题和显示图形
ax.set_title("Randomly Distributed Circles")
plt.show()
阅读全文
相关推荐
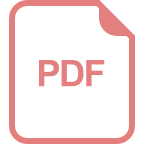
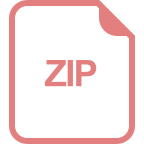
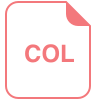
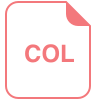










