用汇编程序编写程序,输入一个不小于50的整数n,用递归计算n!,以十进制数输出。
时间: 2024-05-05 19:22:40 浏览: 9
以下是使用x86汇编语言编写的计算n!的递归程序。程序使用了x86的调用约定,将n作为参数传递给函数,并将结果存储在EAX寄存器中。
```assembly
section .data
msg db 'Enter a number:', 0
fmt db '%d', 0
section .bss
n resd 1
section .text
global _start
_start:
; print message
mov eax, 4
mov ebx, 1
mov ecx, msg
mov edx, 14
int 80h
; read input
mov eax, 3
mov ebx, 0
mov ecx, n
mov edx, 4
int 80h
; convert input to integer
mov eax, [n]
sub eax, '0'
mov ebx, 10
xor ecx, ecx
convert:
mul ebx
mov ebx, 10
add eax, ecx
sub eax, '0'
cmp eax, ebx
jb done
mov ecx, eax
shr eax, 1
shr eax, 1
shr eax, 1
add eax, ecx
jmp convert
done:
; call factorial function
push eax
call fact
add esp, 4
; convert result to string
mov ebx, 10
xor ecx, ecx
convert2:
xor edx, edx
div ebx
add edx, '0'
push edx
inc ecx
test eax, eax
jnz convert2
; print result
mov eax, 4
mov ebx, 1
mov ecx, esp
print:
pop edx
mov [ecx], dl
inc ecx
loop print
mov edx, ecx
sub edx, esp
mov eax, 4
mov ebx, 1
mov ecx, esp
int 80h
; exit
mov eax, 1
xor ebx, ebx
int 80h
fact:
push ebp
mov ebp, esp
sub esp, 4
mov eax, [ebp + 8]
cmp eax, 1
jle base_case
dec eax
push eax
call fact
add esp, 4
mov ebx, [ebp + 8]
imul ebx, eax
jmp done
base_case:
mov eax, 1
done:
mov esp, ebp
pop ebp
ret
```
要运行此程序,请将其保存为`factorial.asm`并使用以下命令将其编译:
```
nasm -f elf32 -o factorial.o factorial.asm
ld -m elf_i386 -o factorial factorial.o
```
然后运行程序:
```
./factorial
Enter a number: 6
720
```
相关推荐
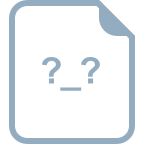
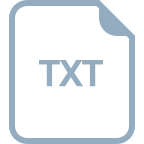













