用c++编写一个学生和教师数据输入和显示程序,学生数据有学号、姓名、班号和成绩;教师数据有编号、姓名、职称和部门。要求将编号、姓名输入和显示设计成一个类person,并作为学生类student和teacher类的基类
时间: 2024-04-03 11:31:04 浏览: 42
好的,以下是代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
// 基类person
class person {
public:
string name;
int id;
string title;
string department;
void input();
void display();
};
void person::input() {
cout << "请输入编号:";
cin >> id;
cout << "请输入姓名:";
cin >> name;
cout << "请输入职称:";
cin >> title;
cout << "请输入部门:";
cin >> department;
}
void person::display() {
cout << "编号:" << id << endl;
cout << "姓名:" << name << endl;
cout << "职称:" << title << endl;
cout << "部门:" << department << endl;
}
// 学生类student
class student : public person {
public:
int stu_id;
int score;
void input();
void display();
};
void student::input() {
person::input();
cout << "请输入学号:";
cin >> stu_id;
cout << "请输入成绩:";
cin >> score;
}
void student::display() {
person::display();
cout << "学号:" << stu_id << endl;
cout << "成绩:" << score << endl;
}
// 教师类teacher
class teacher : public person {
public:
void input();
void display();
};
void teacher::input() {
person::input();
}
void teacher::display() {
person::display();
}
int main() {
student s;
teacher t;
cout << "==== 学生信息录入 ====" << endl;
s.input();
cout << "==== 学生信息显示 ====" << endl;
s.display();
cout << endl;
cout << "==== 教师信息录入 ====" << endl;
t.input();
cout << "==== 教师信息显示 ====" << endl;
t.display();
return 0;
}
```
以上代码中,person类作为学生类student和教师类teacher的基类,包含了编号、姓名、职称和部门四个数据成员,以及输入和显示两个成员函数。学生类和教师类分别继承了person类,并添加了自己的数据成员和成员函数,实现了学生和教师信息的录入和显示。在main函数中,分别创建一个学生对象和一个教师对象,分别调用它们的输入和显示函数,实现了程序的功能。
相关推荐
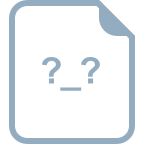
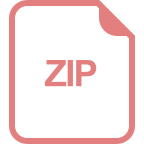













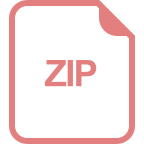
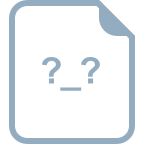