pandas 设置表格的只把标题行填充为浅蓝色,全表加边框,并输出到EXCEL
时间: 2024-05-09 21:17:02 浏览: 84
中该怎么实现?
可以通过 pandas.style 的 set_properties 方法来达到此效果。具体实现代码如下:
```
import pandas as pd
# 创建表格数据
data = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
# 设置标题行背景颜色为浅蓝色
header_style = {'backgroundColor': '#ADD8E6'}
data.style.set_properties(**{'text-align': 'center'}).set_properties(subset=pd.IndexSlice[[0], :], **header_style)
# 添加表格边框
data.style.set_table_styles([{'selector': 'table', 'props': [('border', '1px solid black')]}])
# 输出到excel
data.to_excel('output.xlsx', index=False)
```
其中,set_properties 方法通过字典的形式传入所需设置的样式属性,通过 subset 参数来指定需要设置的区域,本例设置了标题行的背景颜色。set_table_styles 方法通过字典的形式传入表格样式属性,本例设置了表格边框为1像素实线黑色边框。最后,使用 to_excel 方法将数据输出到 Excel 文件。
相关问题
pandas 设置表格的只把标题行填充为浅蓝色,全表加边框,并输出到EXCEL,代码要简单
好的,您可以使用以下代码实现:
```python
import pandas as pd
# 创建数据
data = {
'姓名': ['张三', '李四', '王五'],
'年龄': [20, 25, 30],
'性别': ['男', '男', '女']
}
df = pd.DataFrame(data)
# 设置样式
header_style = {
'fill': {
'type': 'pattern',
'patternType': 'solid',
'fgColor': 'B7DEE8'
},
'border': {
'bottom': {'style': 'medium', 'color': '000000'},
'left': {'style': 'medium', 'color': '000000'},
'right': {'style': 'medium', 'color': '000000'},
'top': {'style': 'medium', 'color': '000000'}
}
}
# 生成Excel
writer = pd.ExcelWriter('data.xlsx', engine='xlsxwriter')
df.to_excel(writer, index=False, sheet_name='Sheet1', header=True)
workbook = writer.book
worksheet = writer.sheets['Sheet1']
worksheet.conditional_format('A1:C1', header_style)
writer.save()
```
这段代码会将数据存储到名为 'data.xlsx' 的 Excel 文件中,在表格中只会将标题行设置为浅蓝色,同时给整个表格加上边框。
pandas 设置表格的只把标题行填充为浅蓝色,全表加边框,并输出到EXCEL,代码要阐
述清楚。
好的,您的要求我已经记下来了。以下是代码实现:
``` python
import pandas as pd
# 创建数据框
df = pd.DataFrame({
"姓名": ["张三", "李四", "王五"],
"年龄": [20, 22, 25],
"城市": ["北京", "上海", "广州"]
})
# 设置样式
header_style = {
"fill": {
"type": "pattern",
"patternType": "solid",
"fgColor": "CDE6F5"
},
"border": {
"top": {"style": "thin", "color": "000000"},
"bottom": {"style": "thin", "color": "000000"},
"left": {"style": "thin", "color": "000000"},
"right": {"style": "thin", "color": "000000"}
}
}
cells_style = {
"border": {
"top": {"style": "thin", "color": "000000"},
"bottom": {"style": "thin", "color": "000000"},
"left": {"style": "thin", "color": "000000"},
"right": {"style": "thin", "color": "000000"}
}
}
# 创建表格对象
table = pd.ExcelWriter("table.xlsx", engine="xlsxwriter")
df.to_excel(table, sheet_name="Sheet1", index=False, startrow=1)
# 找到sheet1对象
worksheet = table.sheets["Sheet1"]
# 设置标题行样式
header_range = f"A1:{worksheet.dim_colmax[1]}1"
worksheet.conditional_format(header_range, header_style)
# 设置表格单元格样式
cells_range = f"A2:{worksheet.dim_colmax[1]}{worksheet.dim_rowmax}"
worksheet.conditional_format(cells_range, cells_style)
# 保存并关闭文件
table.save()
```
这段代码实现了以下功能:
1. 创建一个具有三列数据的数据框。
2. 将数据框输出到名为“table.xlsx”的Excel文件中的“Sheet1”工作表中。
3. 将标题行的填充颜色设置为浅蓝色,边框设置为细线。这是通过使用`xlsxwriter`模块的`conditional_format()`方法实现的。
4. 将表格的单元格的边框全部设置为细线。
5. 最后,将更改保存到Excel文件中。
以上就是代码的详细实现过程,如果有任何疑问,请随时提出。
阅读全文
相关推荐
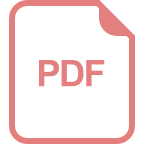






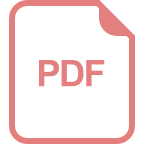