C语言,编写程序,设计函数char * insert(char *s1,char *s2,int n),用指针的方式实现在字符串s1中指定的位置n处插入字符串s2。
时间: 2023-09-24 11:02:40 浏览: 49
可以按照以下方式编写函数`insert`实现在字符串`s1`中指定的位置`n`处插入字符串`s2`:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char *insert(char *s1, char *s2, int n) {
// 计算插入后的字符串长度
int len1 = strlen(s1), len2 = strlen(s2), len = len1 + len2;
// 为插入后的字符串动态分配内存
char *result = (char *)malloc((len + 1) * sizeof(char));
if (result == NULL) {
printf("Memory allocation failed!\n");
exit(1);
}
// 将s1的前n个字符复制到result中
strncpy(result, s1, n);
// 将s2的所有字符复制到result中
strncpy(result + n, s2, len2);
// 将s1的剩余字符复制到result中
strncpy(result + n + len2, s1 + n, len1 - n);
// 在result末尾添加'\0'
result[len] = '\0';
return result;
}
int main() {
char s1[100], s2[100];
int n;
printf("Please enter the string s1: ");
gets(s1);
printf("Please enter the string s2: ");
gets(s2);
printf("Please enter the position to insert: ");
scanf("%d", &n);
char *result = insert(s1, s2, n);
printf("The result string is: %s\n", result);
// 释放动态分配的内存
free(result);
return 0;
}
```
需要注意的是,由于`s1`和`s2`是字符串,其长度可能超过我们定义的数组长度,因此我们使用`gets`函数获取用户输入的字符串,并使用`malloc`函数动态分配内存来存储插入后的字符串。同时,我们还需要在函数结束时释放动态分配的内存,以避免内存泄漏的问题。
相关推荐
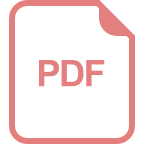
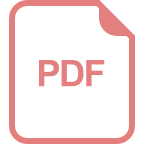














