求10个数里面最大值
时间: 2024-09-18 19:01:50 浏览: 35
求10个数里面的最大值,通常可以采用几种方法:
1. **循环遍历**:创建一个变量(如`max_value`),初始化为第一个数字,然后从第二个数字开始逐个比较,如果当前数大于`max_value`,就更新这个变量。遍历完成后,`max_value`就是最大的数。
```python
numbers = [5, 10, 1, 7, 3, 9, 4, 8, 6, 2]
max_value = numbers[0]
for num in numbers[1:]:
if num > max_value:
max_value = num
max_value
```
2. **内置函数**:某些编程语言提供内置函数可以直接找出最大值,例如Python的`max()`函数,或者JavaScript的`Math.max()`。
```python
numbers = [5, 10, 1, 7, 3, 9, 4, 8, 6, 2]
max_value = max(numbers)
max_value
```
3. **列表排序并取最后一个元素**:将数列排序后,最大的数自然会排在最后一位。
```python
numbers.sort(reverse=True)
max_value = numbers[0]
max_value
```
相关问题
c语言:随机生成10个数,找出10个数里面最大值
在C语言中,你可以使用标准库函数`rand()`和`srand()`来生成随机数,结合数组遍历来找到一组数中的最大值。这里是一个简单的示例程序:
```c
#include <stdio.h>
#include <stdlib.h> // 包含rand()和srand()函数
int main() {
int numbers[10];
int i, max;
// 初始化随机数种子,通常用当前时间
srand(time(0));
// 遍历数组并随机填充元素
for (i = 0; i < 10; ++i) {
numbers[i] = rand() % 100; // 生成0到99之间的随机整数
printf("Generated number: %d\n", numbers[i]);
}
// 找出并打印最大值
max = numbers[0]; // 先假设第一个数是最大的
for (i = 1; i < 10; ++i) {
if (numbers[i] > max) {
max = numbers[i];
}
}
printf("The maximum number is: %d\n", max);
return 0;
}
```
在这个程序中,我们首先初始化了一个包含10个元素的整型数组。然后通过`srand(time(0))`设置随机数种子,使每次运行程序时生成的随机序列不同。接着用循环填充数组元素,并在每次迭代后更新最大值。最后遍历整个数组,找出最大值并将其输出。
c语言:找出10个数里面最大值
在C语言中,你可以使用循环结构和条件判断来找出一组整数中的最大值。下面是一个简单的示例程序,它在一个数组中找到并返回最大的数:
```c
#include <stdio.h>
int findMax(int arr[], int n) {
// 初始化最大值为数组的第一个元素
int max = arr[0];
// 遍历数组,如果当前元素大于已知的最大值,就更新最大值
for (int i = 1; i < n; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
int main() {
int numbers[] = {5, 10, 1, 7, 20, 3, 15, -1, 8, 4};
int size = sizeof(numbers) / sizeof(numbers[0]);
int maxNum = findMax(numbers, size);
printf("数组中最大的数是:%d\n", maxNum);
return 0;
}
```
在这个例子中,`findMax`函数接收一个整数数组和它的大小作为参数,然后通过遍历数组来找出最大值。在`main`函数中,我们创建了一个包含10个数的数组,并调用这个函数获取最大值。
阅读全文
相关推荐
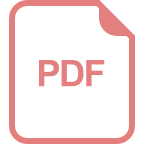
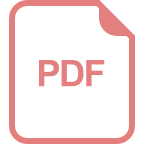
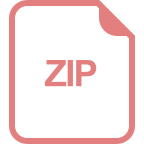













