c++如何判断字符串相等
时间: 2024-11-27 15:21:51 浏览: 20
在 C++ 中,有几种方法可以判断两个字符串是否相等:
1. 使用 `==` 运算符:
```cpp
std::string str1 = "hello";
std::string str2 = "hello";
if (str1 == str2) {
std::cout << "Strings are equal." << std::endl;
}
```
这种方法简单直接,如果两个字符串的内容完全一致,则返回 `true`。
2. 使用 `compare()` 函数:
```cpp
if (str1.compare(str2) == 0) {
// Strings are equal
}
```
`compare()` 函数会比较两个字符串内容,并返回一个整数表示它们的相对位置,如果相等则返回0。
3. 使用 `std::equal()` 和 `std::strlen()`:
```cpp
if (std::equal(str1.begin(), str1.end(), str2.begin()) && strlen(str1.c_str()) == strlen(str2.c_str())) {
// Strings are equal
}
```
这里通过逐字符对比并检查长度是否相等来判断。
相关问题
c++怎么判断字符串相等
在 C++ 中,有多种方法可以判断两个字符串是否相等:
1. 使用 `==` 运算符:这是最直接的方式,比较两个字符串变量的内容是否完全一致。例如:
```cpp
std::string str1 = "hello";
std::string str2 = "hello";
if (str1 == str2) {
std::cout << "两个字符串相等" << std::endl;
}
```
2. 使用 `compare()` 函数:这个函数会返回一个整数,如果两个字符串相等则返回0,不等则返回非零值。例如:
```cpp
if (str1.compare(str2) == 0) {
// 字符串相等
}
```
或者更简洁地写法:
```cpp
if (!str1.compare(str2)) { // 如果不是相等,则compare()会返回false,取反后才是true
// 字符串相等
}
```
3. 使用 `std::equal()` 函数:该函数检查两个迭代器指向的字符是否相等,适用于迭代器遍历整个字符串的情况。
4. 强制转换到 `bool` 类型:由于 `std::string` 可以隐式转换为 `bool` 并表示字符串是否为空,也可以用于判断是否相等:
```cpp
if (str1 == str2 || str1.empty() && str2.empty()) {
// 字符串相等或两者都空
}
```
C++判断字符串是否相等
可以使用C++中的字符串比较函数`strcmp()`来判断两个字符串是否相等。如果返回值为0,则表示两个字符串相等,否则不相等。
示例代码:
```c++
#include <iostream>
#include <cstring>
using namespace std;
int main() {
string str1 = "hello";
string str2 = "world";
string str3 = "hello";
if (strcmp(str1.c_str(), str2.c_str()) == 0) {
cout << "str1 and str2 are equal" << endl;
} else {
cout << "str1 and str2 are not equal" << endl;
}
if (strcmp(str1.c_str(), str3.c_str()) == 0) {
cout << "str1 and str3 are equal" << endl;
} else {
cout << "str1 and str3 are not equal" << endl;
}
return 0;
}
```
输出结果:
```
str1 and str2 are not equal
str1 and str3 are equal
```
阅读全文
相关推荐
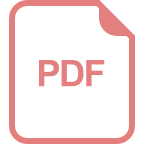
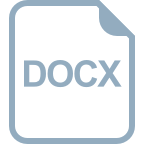
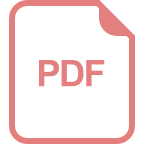













