编写一个函数,将一个字符串中的空格去除,并返回结果串及其首地址,并在main函数中测试。c++
时间: 2024-12-20 22:20:27 浏览: 4
在C++中,你可以创建一个名为`removeSpaces`的函数,它接受一个字符串作为输入,然后使用`std::string`的`erase`方法移除所有的空格。接着,函数会返回处理后的字符串以及新字符串的首地址(即指针)。以下是一个简单的实现:
```cpp
#include <iostream>
#include <string>
// 函数声明,返回值类型为 std::string 和 char*
std::pair<std::string, char*> removeSpaces(const std::string& input);
int main() {
// 测试函数
std::string testString = "Hello World with spaces";
// 调用函数并获取结果
auto result = removeSpaces(testString);
std::string modifiedString = result.first;
char* modifiedStringAddr = &modifiedString[0]; // 将字符数组转换为指针
// 输出结果
std::cout << "Modified string without spaces: " << modifiedString << "\n";
std::cout << "Address of the first character in the modified string: " << modifiedStringAddr << "\n";
return 0;
}
// 实现函数
std::pair<std::string, char*> removeSpaces(const std::string& input) {
std::string cleaned = input.erase(remove(input.begin(), input.end(), ' '), input.end());
return {cleaned, cleaned.c_str()};
}
```
在这个例子中,`removeSpaces`函数首先删除所有空格,然后创建一个新的`std::string`,并将这个新字符串的首地址作为`char*`返回。在`main`函数中,我们打印了修改后的字符串和它的内存地址。
阅读全文
相关推荐
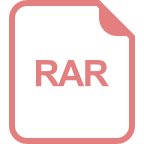
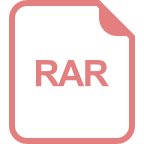
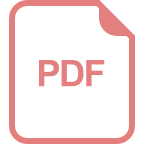















