自定义一个类Apple,实例化出A1 A2 …A5不同重量的5个苹果,如果按照两个苹果的重量相同则判断为相等的话,请重写出equals方法、toString方法及hashCode方法。
时间: 2024-03-24 16:36:45 浏览: 11
好的,我来给你写一个示例代码:
```java
import java.util.Objects;
public class Apple {
private double weight;
public Apple(double weight) {
this.weight = weight;
}
public double getWeight() {
return weight;
}
public void setWeight(double weight) {
this.weight = weight;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Apple apple = (Apple) o;
return Double.compare(apple.weight, weight) == 0;
}
@Override
public int hashCode() {
return Objects.hash(weight);
}
@Override
public String toString() {
return "Apple{" +
"weight=" + weight +
'}';
}
}
```
在这个示例代码中,我们创建了一个Apple类,其中包含一个double类型的weight属性。我们重写了equals方法、toString方法和hashCode方法。
在equals方法中,我们判断如果两个Apple对象的weight属性相同则认为它们是相等的。
在hashCode方法中,我们使用Java 7中引入的Objects.hash方法来计算哈希值。这个方法会根据传入的参数计算出一个哈希值。
在toString方法中,我们返回一个字符串,用来描述Apple对象的属性。
接下来,我们可以使用Apple类来创建5个不同重量的苹果对象:
```java
public class Main {
public static void main(String[] args) {
Apple a1 = new Apple(0.5);
Apple a2 = new Apple(0.6);
Apple a3 = new Apple(0.7);
Apple a4 = new Apple(0.8);
Apple a5 = new Apple(0.9);
System.out.println(a1.equals(a2)); // false
System.out.println(a2.equals(a3)); // false
System.out.println(a3.equals(a4)); // false
System.out.println(a4.equals(a5)); // false
System.out.println(a1.equals(new Apple(0.5))); // true
System.out.println(a1); // Apple{weight=0.5}
System.out.println(a2); // Apple{weight=0.6}
System.out.println(a3); // Apple{weight=0.7}
System.out.println(a4); // Apple{weight=0.8}
System.out.println(a5); // Apple{weight=0.9}
}
}
```
我们可以看到,通过equals方法比较5个苹果对象,只有重量相等的两个苹果对象返回了true。通过toString方法,我们可以很方便地查看每个苹果对象的重量属性。
相关推荐
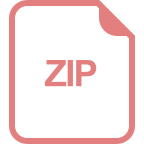
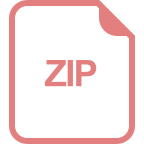












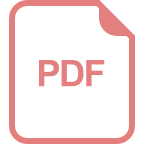
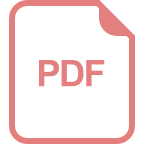