java自定义一个类Apple,实例化出A1 A2 …A5不同重量的5个苹果,如果按照两个苹果的重量相同则判断为相等的话,请重写出equals方法、toString方法及hashCode方法。
时间: 2024-03-18 10:40:30 浏览: 63
好的,我来帮你解答这个问题。
首先,我们定义一个 Apple 类,包含一个 weight 属性表示苹果的重量:
```java
public class Apple {
private int weight;
public Apple(int weight) {
this.weight = weight;
}
// getters and setters
public int getWeight() {
return weight;
}
public void setWeight(int weight) {
this.weight = weight;
}
}
```
接下来,我们需要重写 equals、toString 和 hashCode 方法。由于我们要按照两个苹果的重量相同则判断为相等,所以我们需要在 equals 方法中比较两个苹果的重量是否相同。在 hashCode 方法中,我们将苹果的重量作为哈希值。
```java
public class Apple {
private int weight;
public Apple(int weight) {
this.weight = weight;
}
// getters and setters
public int getWeight() {
return weight;
}
public void setWeight(int weight) {
this.weight = weight;
}
// equals, toString and hashCode
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof Apple)) return false;
Apple apple = (Apple) o;
return weight == apple.weight;
}
@Override
public int hashCode() {
return Objects.hash(weight);
}
@Override
public String toString() {
return "Apple{" +
"weight=" + weight +
'}';
}
}
```
现在,我们可以实例化出 A1, A2, A3, A4, A5 五个不同重量的苹果,并进行比较:
```java
public class Main {
public static void main(String[] args) {
Apple A1 = new Apple(1);
Apple A2 = new Apple(2);
Apple A3 = new Apple(3);
Apple A4 = new Apple(2);
Apple A5 = new Apple(4);
System.out.println(A1.equals(A2)); // false
System.out.println(A2.equals(A3)); // false
System.out.println(A2.equals(A4)); // true
System.out.println(A1.equals(A5)); // false
System.out.println(A1); // Apple{weight=1}
System.out.println(A2); // Apple{weight=2}
System.out.println(A3); // Apple{weight=3}
System.out.println(A4); // Apple{weight=2}
System.out.println(A5); // Apple{weight=4}
}
}
```
注意,我们在重写 equals 方法时需要判断传入的对象是否为 Apple 类型,这可以通过使用 instanceof 关键字来实现。同时,我们还需要在 hashCode 方法中使用 Objects.hash 方法来计算哈希值。最后,我们可以通过调用 System.out.println 方法来打印苹果的信息。
阅读全文
相关推荐
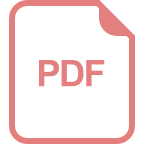
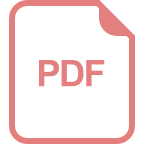

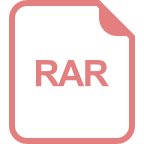
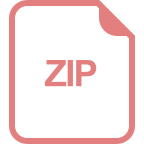
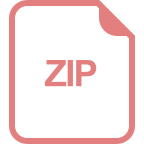
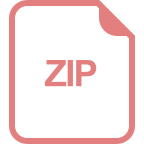
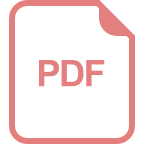
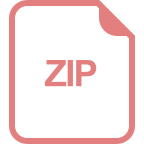
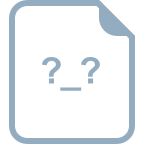
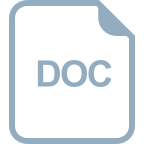
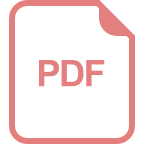
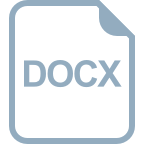
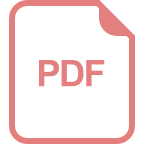
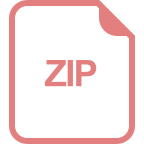