openmv与msp430f5529循黑色线小车代码
时间: 2023-08-03 15:05:10 浏览: 90
您好!很高兴回答您的问题。以下是一个示例代码,演示如何使用OpenMV和MSP430F5529控制循迹小车。
首先,我们需要在OpenMV上编写代码来检测黑色线。以下是一个基本的示例:
```python
import sensor
import image
import time
from pyb import UART
# 初始化串口
uart = UART(3, 115200)
# 初始化摄像头
sensor.reset()
sensor.set_pixformat(sensor.GRAYSCALE)
sensor.set_framesize(sensor.QQVGA)
sensor.skip_frames(time=2000)
# 设置阈值来区分黑线和白色背景
GRAYSCALE_THRESHOLD = (0, 100)
while(True):
img = sensor.snapshot()
# 通过二值化图像来检测黑线
img.binary([GRAYSCALE_THRESHOLD])
# 通过find_blobs函数找到黑线的区域
blobs = img.find_blobs([GRAYSCALE_THRESHOLD], pixels_threshold=200, area_threshold=200)
if blobs:
# 找到最大面积的区域
max_blob = max(blobs, key=lambda b: b.pixels())
# 在图像上画出线的位置
img.draw_rectangle(max_blob.rect())
img.draw_cross(max_blob.cx(), max_blob.cy())
# 发送线的位置给MSP430F5529
uart.write("%d\n" % max_blob.cx())
```
接下来,我们需要在MSP430F5529上编写代码来接收OpenMV发送的线的位置,并控制小车循迹。以下是一个简单的示例:
```c
#include <msp430.h>
#include <stdio.h>
#include <stdlib.h>
#define RX_BUFFER_SIZE 10
volatile char rx_buffer[RX_BUFFER_SIZE];
volatile unsigned int rx_index = 0;
volatile unsigned int line_position = 0;
void uart_init()
{
P4SEL |= BIT4 + BIT5;
UCA1CTL1 |= UCSWRST;
UCA1CTL1 |= UCSSEL_2;
UCA1BR0 = 6; // 9600 baud rate
UCA1BR1 = 0;
UCA1MCTL |= UCBRS_0 + UCBRF_13 + UCOS16;
UCA1CTL1 &= ~UCSWRST;
UCA1IE |= UCRXIE;
}
void main(void)
{
WDTCTL = WDTPW + WDTHOLD;
uart_init();
while (1)
{
// 根据线的位置控制小车运动
if (line_position < 80)
{
// 左转
P1DIR |= BIT2 + BIT3;
P1OUT &= ~BIT2;
P1OUT |= BIT3;
}
else if (line_position > 160)
{
// 右转
P1DIR |= BIT2 + BIT3;
P1OUT |= BIT2;
P1OUT &= ~BIT3;
}
else
{
// 直行
P1DIR &= ~(BIT2 + BIT3);
P1OUT |= BIT2 + BIT3;
}
}
}
#pragma vector=USCI_A1_VECTOR
__interrupt void USCI_A1_ISR(void)
{
switch (__even_in_range(UCA1IV, 4))
{
case 0:
break;
case 2:
rx_buffer[rx_index++] = UCA1RXBUF;
if (rx_buffer[rx_index - 1] == '\n')
{
line_position = atoi(rx_buffer);
rx_index = 0;
}
break;
default:
break;
}
}
```
这是一个简单的示例,仅供参考。您可能需要根据您的具体硬件和需求进行适当的修改。
希望对您有所帮助!如有任何疑问,请随时提问。
相关推荐
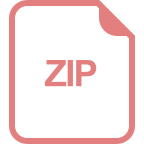














