用Python编写代码,实现基于Hough变换的“矩形矫正”,和瓶子盖轮廓提取。
时间: 2024-11-04 10:11:57 浏览: 45
在Python中,我们可以使用OpenCV库来实现基于霍夫变换(Hough Transform)的矩形矫正和瓶子盖轮廓提取。这里是一个简化的示例,展示如何进行这两个步骤:
首先,导入必要的库:
```python
import cv2
import numpy as np
```
**1. 矩形矫正 (Hough Transformation)**
假设我们有一个图像 `img`,并且想找到图像中最接近正方形的矩形。可以按照以下步骤进行:
```python
# 预处理图像:灰度化、二值化、边缘检测
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
_, binary = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
edges = cv2.Canny(binary, low_threshold=50, high_threshold=150)
# 使用霍夫变换寻找近似正方形
lines = cv2.HoughLinesP(edges, rho=1, theta=np.pi/180, threshold=40, minLineLength=100, maxLineGap=10)
# 从线条中选择最接近正方形的
best_square_lines = []
for line in lines:
x1, y1, x2, y2 = line[0]
angle = np.arctan2(y2 - y1, x2 - x1)
if abs(angle - np.pi / 4) < abs(angle - 3 * np.pi / 4): # 更接近90度
best_square_lines.append(line)
if len(best_square_lines) > 0:
# 画出选定的直线并找到矩形中心
rect_lines = np.sum(best_square_lines, axis=0)
x, y, w, h = cv2.boundingRect(rect_lines)
# 使用该矩形对原图进行纠正
corrected_img = img[y:y+h, x:x+w]
else:
print("No suitable rectangle found.")
```
**2. 瓶子盖轮廓提取**
对于瓶子盖的轮廓提取,我们需要先确定感兴趣区域(ROI),然后应用轮廓检测算法:
```python
# ROI (例如,通过上面找到的矩形)
crop_img = corrected_img
# 转换成二进制
_, crop_binary = cv2.threshold(crop_img, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
# 寻找轮廓
contours, _ = cv2.findContours(crop_binary, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 找到面积最大的轮廓作为瓶子盖候选
max_contour_area = 0
max_contour = None
for contour in contours:
area = cv2.contourArea(contour)
if area > max_contour_area:
max_contour_area = area
max_contour = contour
if max_contour is not None:
# 根据轮廓绘制边界框
x, y, w, h = cv2.boundingRect(max_contour)
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 255, 0), 2)
else:
print("No bottle cap contour detected.")
阅读全文
相关推荐







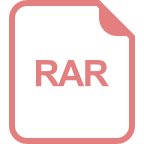


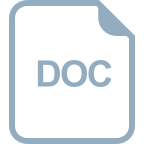

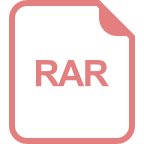


