请用Python编写代码,实现基于Hough变换的“矩形矫正”
时间: 2024-11-10 07:14:40 浏览: 36
在Python中,我们可以使用OpenCV库来实现基于霍夫变换(Hough Transform)的矩形检测和矫正功能。首先,你需要安装`opencv-python`库,如果没有安装,可以使用pip install opencv-python命令进行安装。
下面是一个简单的例子,展示了如何通过霍夫变换找到图像中最接近矩形的直线,并进一步重构矩形:
```python
import cv2
import numpy as np
def detect_rectangle(image_path):
# 读取图像
img = cv2.imread(image_path)
# 转为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 高斯滤波减少噪声
blurred = cv2.GaussianBlur(gray, (5, 5), 0)
# 计算边缘
edges = cv2.Canny(blurred, 50, 150)
# 构建投票板,每个单元格代表一条可能的直线
rho = 1 # 线段长度步长
theta = np.pi / 180 # 角度间隔
threshold = 10 # 需要有多少投票才能确认线段
min_line_length = 100 # 最小线段长度
max_line_gap = 20 # 连接线段的最大间隙
lines = cv2.HoughLinesP(edges, rho, theta, threshold, np.array([]),
minLineLength=min_line_length,
maxLineGap=max_line_gap)
# 根据直线找到可能的矩形框
rectangles = []
for line in lines:
x1, y1, x2, y2 = line[0]
if abs(y2 - y1) > abs(x2 - x1): # 优先考虑水平和垂直方向
continue
if x1 < x2: # 只保留正确的矩形方向
rectangles.append(((x1, y1), (x2, y2)))
# 使用透视变换矫正矩形
if rectangles:
rectangle = rectangles[0] # 我们假设只有一个矩形
src_points = np.float32(rectangle).reshape(-1, 1, 2)
dst_points = np.float32([[0, 0], [img.shape[1]-1, 0],
[img.shape[1]-1, img.shape[0]-1], [0, img.shape[0]-1]])
M = cv2.getPerspectiveTransform(src_points, dst_points)
warped_img = cv2.warpPerspective(img, M, (img.shape[1], img.shape[0]))
return warped_img
# 使用函数并保存结果
warped_image = detect_rectangle('input_image.jpg')
cv2.imwrite('output_warped_image.jpg', warped_image)
```
在这个代码中,我们首先检测到图像中的边缘,然后寻找最有可能构成矩形的直线。接着,我们根据这些直线构建透视变换矩阵,最后对原始图像应用透视变换以得到矫正后的矩形。
注意:这个代码示例假设图片中存在一个矩形区域,并尝试对其进行矫正。实际应用中,你可能需要对输入的图片和参数进行一些调整,例如处理复杂场景下的多个矩形或多边形。
阅读全文
相关推荐
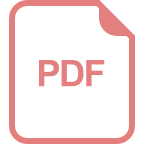
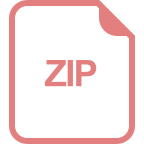
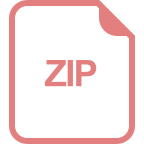



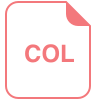
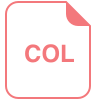
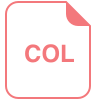


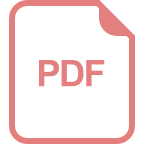
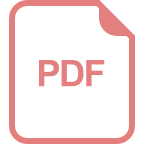
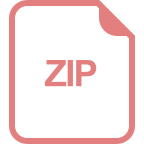